資源:Youtube Channel – freeCodeCamp.org
教師:Kevin Powell, Thank you
影片時間:04:11:03
Course content
Intro
1. Starting to think responsively
Building websites used to be simple. We had a single design, we made the site look exactly like that design, and we were done.
Now we must make a design and have it work on every device, from a watch screen to a 80″ TV.
Now that we have the fundamentals down, it’s time to get into the responsive frame of mind.
We’ll work our way up from getting a design to simply work on a single screen size to being able to get it to work on any device.
Thinking responsively isn’t easy, especially at first.
We’ll work our way up, exploring the do’s and don’ts and how to plan things out to make your life easier in the long run.
This is just an intro thinking responsively.
We won’t be covering everything, but this is to set the stage for everything that comes after it.
What we’ll be covering in this module:
- How to approach a layout
- CSS units (absolute, relative, and percentages)
- Flexbox basics (our layout tool)
- Media query basics (adapting to different screen sizes)
2. CSS Units
CSS Units
Starting to think responsively
There are different types of units that we can use
CSS units come in a few different flavors:
- Absolute units
- Relative units
- Percentage
CSS Units
Absolute units are the easiest to understand.
- Pixels (px)
- pt, cm, mm, in, etc
CSS Units – Percentage
Percentages are mainly used for widths, and are pretty easy to understand.
- Relative to their parent (except on height, where things get weird).
CSS Units – Relative units
There are two types of relative units.
- Relative to font-size
- Relative to the viewport
Even though it sounds complicated, once you play around with them a little it isn’t too bad!
CSS Units – Relative units
- Relative to font-size:
- em and rem (and many other less common ones)
- Relative to the viewport (we’ll get to this later)
- vw, vh, vmin, vmax
3. CSS Units – Percentage
Percentages
- 寬度 – 960px
- 左邊 – 960 * 0.7 = 672px
- And then there is the other side
- And what about the padding?
- And that’s for a fixed width!
- We need to think responsively
程式碼範例 – .container
width: 620px; → width: 50%;
.container {
width: 50%;
margin: 0 auto;
}
4. Controlling the width of images
程式碼範例 – img
img {
width: 100%;
}
width: 50%; → width: 90%;
.container {
width: 90%;
margin: 0 auto;
}
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>intro to rwd</title>
<link rel="stylesheet" href="css/all.css">
</head>
<body>
<header>
<div class="container">
<h1>程序員那麼可愛</h1>
</div>
</header>
<section class="section-one">
<div class="container">
<h2>關於愛情</h2>
<img src="images/banner.jpg" alt="banner jpg">
<ul>
<li>EP1.暗戀就像是等待測試的程序,不確定運行結果,也不確定運行時間,但只要需求沒變,就要努力優化程序,直到它成功運行。</li>
<li>EP2.喜歡你這件事,不會有任何BUG,所以不需要DEBUG。</li>
<li>EP3.和暗戀的人在一起時,記得敲一遍高精度,這樣對他的喜歡,就不會溢出,被對方發現了。</li>
<li>EP4.每個人都有屬於自己的秘密,雖然可以通過技術手段進行加密,但卻不知道系統何時會被黑客入侵,將我們保守的秘密公之於眾。</li>
<li>EP5.COMPUTERS NEVER LIE,人的內心也同計算機一樣不會說謊,只是人們常常忽略了,心底最真實的想法。</li>
</ul>
</div>
</section>
<section class="section-two">
<div class="container">
<h2>劇情介紹</h2>
<p>陸漓(<a href="#">祝緒丹</a>飾)是女程式設計師。為了追求理想而投身編程領域,她憑藉自身經歷及過人的智慧搞定學長姜逸城(<a href="#">邢昭林</a>飾),擺平他無數次的相親後,成功進入異承公司,勇闖IT世界。倆人因程序代碼結緣,後在機緣巧合下成為同居的室友。性格迥異的他們在同居相處中鬥智鬥勇情況下,是否會意外觸發心動的代碼?</p>
<a href="#" class="btn btn-official">連結到官方微博</a>
<a href="#" class="btn btn-white">更多花絮</a>
</div>
</section>
</body>
</html>
CSS
body{
margin: 0;
font-size: 18px;
font-family: 'Lato', sans-serif;
color: #2e354f;
}
img{
width: 100%;
}
h1,
h2,
strong{
font-weight: 900;
}
/* Typography */
h1{
font-size: 36px;
margin: 0;
}
h2{
font-size: 24px;
color: #ef5839;
}
a{
color: #ef5839;
}
a:hover,
a:focus{
color: #d4b44c;
}
/* buttons */
.btn{
display: inline-block;
padding: 10px 25px;
text-decoration: none;
}
.btn-official{
background: #ef5839;
color: #fff;
}
.btn-official:hover{
color: #2e354f;
background: #d4b44c;
}
.btn-white{
background: #fff;
color: #2e35ff;
}
.btn-white:hover{
color: #2e354f;
background: #999;
}
/* Layout */
header{
background: #2e354f;
padding: 45px 0;
color: #fff;
}
section{
padding: 45px 0
}
.container{
width: 90%;
margin: 0 auto;
}
.section-two{
background: #2e354f;
color: #fff;
}
5. min-width and max-width
.container {
width: 90%;
max-width: 620px;
border: 2px solid magenta;
margin: 0 auto;
}
6. CSS Units – The em unit
The “em” unit
Starting to think responsively
CSS Units – Relative units
Relative units are always relative either to a font-size, or the size of the viewport.
For those which are relative to a font-size, which font-size they are relative to depends on a few things, which complicates matters.
CSS Units – Relative units
The em and rem are considered relative, because they are relative to the font-size of other elements
- em are relative to their parent’s font-size
- Font-size is an inherited property, so if you don’t declare it anywhere, it’s getting it from the body (or the default, which is normally 16px)
- Let’s see how they work
程式碼範例
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>intro to rwd</title>
<link rel="stylesheet" href="css/all.css">
</head>
<body>
<header>
<div class="container">
<h1>程序員那麼可愛</h1>
</div>
</header>
<section class="section-one">
<div class="container">
<h2>關於愛情</h2>
<img src="images/banner.jpg" alt="banner jpg">
<ul>
<li>EP1.暗戀就像是等待測試的程序,不確定運行結果,也不確定運行時間,但只要需求沒變,就要努力優化程序,直到它成功運行。</li>
<li>EP2.喜歡你這件事,不會有任何BUG,所以不需要DEBUG。</li>
<li>EP3.和暗戀的人在一起時,記得敲一遍高精度,這樣對他的喜歡,就不會溢出,被對方發現了。</li>
<li>EP4.每個人都有屬於自己的秘密,雖然可以通過技術手段進行加密,但卻不知道系統何時會被黑客入侵,將我們保守的秘密公之於眾。</li>
<li>EP5.COMPUTERS NEVER LIE,人的內心也同計算機一樣不會說謊,只是人們常常忽略了,心底最真實的想法。</li>
</ul>
</div>
</section>
<section class="section-two">
<div class="container">
<h2>劇情介紹</h2>
<p>陸漓(<a href="#">祝緒丹</a>飾)是女程式設計師。為了追求理想而投身編程領域,她憑藉自身經歷及過人的智慧搞定學長姜逸城(<a href="#">邢昭林</a>飾),擺平他無數次的相親後,成功進入異承公司,勇闖IT世界。倆人因程序代碼結緣,後在機緣巧合下成為同居的室友。性格迥異的他們在同居相處中鬥智鬥勇情況下,是否會意外觸發心動的代碼?</p>
<a href="#" class="btn btn-official">連結到官方微博</a>
<a href="#" class="btn btn-white">更多花絮</a>
</div>
</section>
</body>
</html>
CSS
body{
margin: 0;
font-size: 16px;
font-family: 'Lato', sans-serif;
color: #2e354f;
}
.section-one{
/* font-size: 20px; */
}
ul{
/* 1.5em = 150% */
font-size: 1.5em;
}
img{
width: 100%;
}
h1,
h2,
strong{
font-weight: 900;
}
/* Typography */
h1{
font-size: 3em;
margin: 0;
}
h2{
font-size: 2em;
color: #ef5839;
}
a{
color: #ef5839;
}
a:hover,
a:focus{
color: #d4b44c;
}
/* buttons */
.btn{
display: inline-block;
padding: 10px 25px;
text-decoration: none;
}
.btn-official{
background: #ef5839;
color: #fff;
}
.btn-official:hover{
color: #2e354f;
background: #d4b44c;
}
.btn-white{
background: #fff;
color: #2e35ff;
}
.btn-white:hover{
color: #2e354f;
background: #999;
}
/* Layout */
header{
background: #2e354f;
padding: 45px 0;
color: #fff;
}
section{
padding: 45px 0
}
.container{
width: 90%;
max-width: 620px;
margin: 0 auto;
border: 2px solid magenta;
}
.section-two{
background: #2e354f;
color: #fff;
}
7. The problem with ems
CSS Units – The problem with em
em units are very convenient for some things, but…
When we use them for the font-size of an element though, it can create a cascading effect.
程式碼範例
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>intro to rwd</title>
<link rel="stylesheet" href="css/all.css">
</head>
<body>
<header>
<div class="container">
<h1>程序員那麼可愛</h1>
</div>
</header>
<section class="section-one">
<div class="container">
<h2>關於愛情</h2>
<img src="images/banner.jpg" alt="banner jpg">
<ul>
<li>EP1.暗戀就像是等待測試的程序,不確定運行結果,也不確定運行時間,但只要需求沒變,就要努力優化程序,直到它成功運行。</li>
<li>EP2.喜歡你這件事,不會有任何BUG,所以不需要DEBUG。</li>
<li>EP3.和暗戀的人在一起時,記得敲一遍高精度,這樣對他的喜歡,就不會溢出,被對方發現了。</li>
<li>EP4.每個人都有屬於自己的秘密,雖然可以通過技術手段進行加密,但卻不知道系統何時會被黑客入侵,將我們保守的秘密公之於眾。</li>
<li>EP5.COMPUTERS NEVER LIE,人的內心也同計算機一樣不會說謊,只是人們常常忽略了,心底最真實的想法。</li>
</ul>
</div>
</section>
<section class="section-two">
<div class="container">
<h2>劇情介紹</h2>
<p>陸漓(<a href="#">祝緒丹</a>飾)是女程式設計師。為了追求理想而投身編程領域,她憑藉自身經歷及過人的智慧搞定學長姜逸城(<a href="#">邢昭林</a>飾),擺平他無數次的相親後,成功進入異承公司,勇闖IT世界。倆人因程序代碼結緣,後在機緣巧合下成為同居的室友。性格迥異的他們在同居相處中鬥智鬥勇情況下,是否會意外觸發心動的代碼?</p>
<a href="#" class="btn btn-official">連結到官方微博</a>
<a href="#" class="btn btn-white">更多花絮</a>
</div>
</section>
</body>
</html>
CSS
body{
margin: 0;
font-size: 10px;
font-family: 'Lato', sans-serif;
color: #2e354f;
}
.section-one{
font-size: 2em;
}
ul{
/* 1.5em = 150% */
font-size: 1.5em;
}
li{
font-size: 1.5em;
}
img{
width: 100%;
}
h1,
h2,
strong{
font-weight: 900;
}
/* Typography */
h1{
font-size: 3em;
margin: 0;
}
h2{
font-size: 2em;
color: #ef5839;
}
a{
color: #ef5839;
}
a:hover,
a:focus{
color: #d4b44c;
}
/* buttons */
.btn{
display: inline-block;
padding: 10px 25px;
text-decoration: none;
}
.btn-official{
background: #ef5839;
color: #fff;
}
.btn-official:hover{
color: #2e354f;
background: #d4b44c;
}
.btn-white{
background: #fff;
color: #2e35ff;
}
.btn-white:hover{
color: #2e354f;
background: #999;
}
/* Layout */
header{
background: #2e354f;
padding: 45px 0;
color: #fff;
}
section{
padding: 45px 0
}
.container{
width: 90%;
max-width: 620px;
margin: 0 auto;
border: 2px solid magenta;
}
.section-two{
background: #2e354f;
color: #fff;
}
8. The Solution: Rems
The solution: rems
Starting to think responsively
CSS Units – The problem with em
The rem unit is short for Root Em.
That means it’s always relative to the “root” of our document.
The root of an HTML page is always the html element.
程式碼範例
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>intro to rwd</title>
<link rel="stylesheet" href="css/all.css">
</head>
<body>
<header>
<div class="container">
<h1>程序員那麼可愛</h1>
</div>
</header>
<section class="section-one">
<div class="container">
<h2>關於愛情</h2>
<img src="images/banner.jpg" alt="banner jpg">
<ul>
<li>EP1.暗戀就像是等待測試的程序,不確定運行結果,也不確定運行時間,但只要需求沒變,就要努力優化程序,直到它成功運行。</li>
<li>EP2.喜歡你這件事,不會有任何BUG,所以不需要DEBUG。</li>
<li>EP3.和暗戀的人在一起時,記得敲一遍高精度,這樣對他的喜歡,就不會溢出,被對方發現了。</li>
<li>EP4.每個人都有屬於自己的秘密,雖然可以通過技術手段進行加密,但卻不知道系統何時會被黑客入侵,將我們保守的秘密公之於眾。</li>
<li>EP5.COMPUTERS NEVER LIE,人的內心也同計算機一樣不會說謊,只是人們常常忽略了,心底最真實的想法。</li>
</ul>
</div>
</section>
<section class="section-two">
<div class="container">
<h2>劇情介紹</h2>
<p>陸漓(<a href="#">祝緒丹</a>飾)是女程式設計師。為了追求理想而投身編程領域,她憑藉自身經歷及過人的智慧搞定學長姜逸城(<a href="#">邢昭林</a>飾),擺平他無數次的相親後,成功進入異承公司,勇闖IT世界。倆人因程序代碼結緣,後在機緣巧合下成為同居的室友。性格迥異的他們在同居相處中鬥智鬥勇情況下,是否會意外觸發心動的代碼?</p>
<a href="#" class="btn btn-official">連結到官方微博</a>
<a href="#" class="btn btn-white">更多花絮</a>
</div>
</section>
</body>
</html>
CSS – 62.5% → 625% (10px → 100px)
因為開發常用的Chrome瀏覽器,支援的最小字體是12px,所以要改為625%
html{
font-size: 625%;
}
body{
margin: 0;
font-size: 0.1rem;
font-family: 'Lato', sans-serif;
color: #2e354f;
}
.section-one{
font-size: 0.2rem;
}
ul{
font-size: 0.15rem;
}
img{
width: 100%;
}
h1,
h2,
strong{
font-weight: 900;
}
/* Typography */
h1{
font-size: 0.3rem;
margin: 0;
}
h2{
font-size: 0.2rem;
color: #ef5839;
}
a{
color: #ef5839;
}
a:hover,
a:focus{
color: #d4b44c;
}
/* buttons */
.btn{
display: inline-block;
padding: 10px 25px;
text-decoration: none;
}
.btn-official{
background: #ef5839;
color: #fff;
}
.btn-official:hover{
color: #2e354f;
background: #d4b44c;
}
.btn-white{
background: #fff;
color: #2e35ff;
}
.btn-white:hover{
color: #2e354f;
background: #999;
}
/* Layout */
header{
background: #2e354f;
padding: 45px 0;
color: #fff;
}
section{
padding: 45px 0
}
.container{
width: 90%;
max-width: 620px;
margin: 0 auto;
border: 2px solid magenta;
}
.section-two{
background: #2e354f;
color: #fff;
}
9. Picking which unit to use
How to decide which unit to use
Starting to think responsively
How to decide which unit to use
Pixels used to cause a lot of problems, as they were a fixed unit (one dot on the screen).
Now, it follows the reference pixel.
How to decide which unit to use
That also makes my answer a little less concrete than I use to have.
But there are advantages to using rem and em.
How to decide which unit to use
So I’m going to stick with those.
My general rule of thumb:
- Font-size = rem
- Padding and margin = rem
- Widths = em or percentage
- Those are a “rule of thumb”, not hard and fast rules
- Let’s see how they work
10. ems and rems – an example
程式碼範例
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>intro to rwd</title>
<link rel="stylesheet" href="css/all.css">
</head>
<body>
<div class="card">
<h1><span class="accent-color">程序員</span>那麼可愛</h1>
<p>EP1.暗戀就像是等待測試的程序,不確定運行結果,也不確定運行時間,但只要需求沒變,就要努力優化程序,直到它成功運行。</p>
<p>EP2.喜歡你這件事,不會有任何<a>BUG</a>,所以不需要<strong>DEBUG</strong>。</p>
<p>EP3.和暗戀的人在一起時,記得敲一遍高精度,這樣對他的喜歡,就不會溢出,被對方發現了。</p>
<p class="links">
<a class="button btn-big" href="#">連結到官方微博</a>
<a class="button" href="#">回首頁</a>
<a href="#" class="button btn-small">更多資訊</a>
</p>
</div>
</body>
</html>
CSS
.card{
background: #000;
color: #fff;
width: 560px;
margin: 0 auto;
padding: 45px;
}
h1, p{
margin-top: 0;
}
h1{
font-size: 3rem;
margin-bottom: 2em;
}
p{
font-size: 18px;
}
a{
color: #000;
}
.button{
display: inline-block;
background: #ffe600;
color: #000;
padding: 0.5em 1.25em;
/* em is relative to the font-size of this element */
text-align: center;
text-decoration: none;
margin-bottom: 50px;
}
.button:hover{
background: orange;
}
.btn-big{
font-size: 1.5rem;
}
.btn-small{
font-size: 0.75rem;
}
.accent-color{
color: #ffe600;
}
.links{
margin-top: 0;
margin-bottom: 0;
}
11. Flexbox refresher and setting up some HTML
Responsive layout basics
Starting to think responsively
The basics of flexbox
Elements normally have a display: block or a display: inline as a default from the browser.
投影片範例
CSS
.parent {
display: flex;
}
The basics of flexbox
Block elements stack on the top of the each other
- div, header, footer, main, etc
- h1 → h6
- p
The basics of flexbox
Inline elements stay within the flow of what’s around them
- a
- strong
- em
- span
The basics of flexbox
We can change this behavior by setting the display property to flex on the parent element.
網站結構
- .container
- .columns
- .col
- .col
- .col
- .columns
- .col
- .col
- .columns
程式碼範例
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>intro to rwd</title>
<link rel="stylesheet" href="css/all.css">
</head>
<body>
<div class="container">
<h1>程序員那麼可愛</h1>
<img src="images/banner.jpg" alt="banner jpg">
<div class="columns">
<div class="col">
<h2>關於愛情</h2>
<p></p>
</div>
<div class="col">
<h2></h2>
<p></p>
<p></p>
</div>
<div class="col">
<h2></h2>
<p></p>
</div>
</div>
<div class="columns">
<div class="col">
<h2></h2>
<p></p>
</div>
<div class="col">
<p></p>
</div>
</div>
</div>
</body>
</html>
12. Basic Styles and setting up the columns
Colors
- #312614
- #B7832F
- #707070
- #FFF
Font-sizes
- h1: 3rem
- h2: 1.5rem
- body: 1.125rem
Layout
- max-width: 980px
程式碼範例
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>intro to rwd</title>
<link rel="stylesheet" href="css/all.css">
</head>
<body>
<div class="container">
<h1>程序員那麼<span>可愛</span></h1>
<img src="images/banner.jpg" alt="banner jpg">
<div class="columns">
<div class="col">
<h2>關於愛情 EP01</h2>
<p>暗戀就像是等待測試的程序,不確定運行結果,也不確定運行時間,但只要需求沒變,就要努力優化程序,直到它成功運行。</p>
</div>
<div class="col">
<h2>關於愛情 EP02、EP03</h2>
<p>喜歡你這件事,不會有任何BUG,所以不需要DEBUG。</p>
<p>和暗戀的人在一起時,記得敲一遍高精度,這樣對他的喜歡,就不會溢出,被對方發現了。</p>
</div>
<div class="col">
<p>劇情簡介:陸漓(祝緒丹飾)是女程式設計師。為了追求理想而投身編程領域,她憑藉自身經歷及過人的智慧搞定學長姜逸城(邢昭林飾),擺平他無數次的相親後,成功進入異承公司,勇闖IT世界。倆人因程序代碼結緣,後在機緣巧合下成為同居的室友。性格迥異的他們在同居相處中鬥智鬥勇情況下,是否會意外觸發心動的代碼?</p>
</div>
</div>
<div class="columns">
<div class="col">
<h2>關於愛情 EP04</h2>
<p>每個人都有屬於自己的秘密,雖然可以通過技術手段進行加密,但卻不知道系統何時會被黑客入侵,將我們保守的秘密公之於眾。</p>
</div>
<div class="col">
<p>導語:為了自己的暗戀事業和人生目標,陸漓拼盡一切抓住機會,終於和姜逸城達成一年的“契約夫婦”。兩人在同居中展開甜蜜互動,姜逸城對陸漓漸漸動心而不自知……</p>
</div>
</div>
</div>
</body>
</html>
CSS
body {
font-size: 1.125rem;
color: #707070;
margin: 0;
}
/* Typography */
h1 {
font-size: 3rem;
color: #212614;
text-align: center;
}
h1 span{
color: #b7832f;
}
h2 {
font-size: 1.5rem;
}
/* Layout */
img {
max-width: 100%;
}
.container {
width: 95%;
max-width: 980px;
margin: 0 auto;
}
.columns {
display: flex;
}
13. Adding the background color
程式碼範例
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>intro to rwd</title>
<link rel="stylesheet" href="css/all.css">
</head>
<body>
<div class="container">
<h1>程序員那麼<span>可愛</span></h1>
<img src="images/banner.jpg" alt="banner jpg">
<div class="columns">
<div class="col">
<h2>關於愛情 EP01</h2>
<p>暗戀就像是等待測試的程序,不確定運行結果,也不確定運行時間,但只要需求沒變,就要努力優化程序,直到它成功運行。</p>
</div>
<div class="col">
<h2>關於愛情 EP02、EP03</h2>
<p>喜歡你這件事,不會有任何BUG,所以不需要DEBUG。</p>
<p>和暗戀的人在一起時,記得敲一遍高精度,這樣對他的喜歡,就不會溢出,被對方發現了。</p>
</div>
<div class="col col-bg">
<p>劇情簡介:陸漓(祝緒丹飾)是女程式設計師。為了追求理想而投身編程領域,她憑藉自身經歷及過人的智慧搞定學長姜逸城(邢昭林飾),擺平他無數次的相親後,成功進入異承公司,勇闖IT世界。倆人因程序代碼結緣,後在機緣巧合下成為同居的室友。性格迥異的他們在同居相處中鬥智鬥勇情況下,是否會意外觸發心動的代碼?</p>
</div>
</div>
<div class="columns">
<div class="col">
<h2>關於愛情 EP04</h2>
<p>每個人都有屬於自己的秘密,雖然可以通過技術手段進行加密,但卻不知道系統何時會被黑客入侵,將我們保守的秘密公之於眾。</p>
</div>
<div class="col col-bg">
<p>導語:為了自己的暗戀事業和人生目標,陸漓拼盡一切抓住機會,終於和姜逸城達成一年的“契約夫婦”。兩人在同居中展開甜蜜互動,姜逸城對陸漓漸漸動心而不自知……</p>
</div>
</div>
</div>
</body>
</html>
CSS
body {
font-size: 1.125rem;
color: #707070;
margin: 0;
}
/* Typography */
h1 {
font-size: 3rem;
color: #212614;
text-align: center;
}
h1 span{
color: #b7832f;
}
h2 {
font-size: 1.5rem;
}
p {
margin-top: 0;
}
/* Layout */
img {
max-width: 100%;
}
.container {
width: 95%;
max-width: 980px;
margin: 0 auto;
}
.columns {
display: flex;
margin: 1em 0;
}
.col-bg{
background: #212614;
color: #fff;
padding: 1em;
}
14. Setting the column widths
程式碼範例
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>intro to rwd</title>
<link rel="stylesheet" href="css/all.css">
</head>
<body>
<div class="container">
<h1>程序員那麼<span>可愛</span></h1>
<img src="images/banner.jpg" alt="banner jpg">
<div class="columns">
<div class="col col-1">
<h2>關於愛情 EP01</h2>
<p>暗戀就像是等待測試的程序,不確定運行結果,也不確定運行時間,但只要需求沒變,就要努力優化程序,直到它成功運行。</p>
</div>
<div class="col col-2">
<h2>關於愛情 EP02、EP03</h2>
<p>喜歡你這件事,不會有任何BUG,所以不需要DEBUG。</p>
<p>和暗戀的人在一起時,記得敲一遍高精度,這樣對他的喜歡,就不會溢出,被對方發現了。</p>
</div>
<div class="col col-1 col-bg">
<p>劇情簡介:陸漓(祝緒丹飾)是女程式設計師。為了追求理想而投身編程領域,她憑藉自身經歷及過人的智慧搞定學長姜逸城(邢昭林飾),擺平他無數次的相親後,成功進入異承公司,勇闖IT世界。倆人因程序代碼結緣,後在機緣巧合下成為同居的室友。性格迥異的他們在同居相處中鬥智鬥勇情況下,是否會意外觸發心動的代碼?</p>
</div>
</div>
<div class="columns">
<div class="col col-3">
<h2>關於愛情 EP04</h2>
<p>每個人都有屬於自己的秘密,雖然可以通過技術手段進行加密,但卻不知道系統何時會被黑客入侵,將我們保守的秘密公之於眾。</p>
</div>
<div class="col col-1 col-bg">
<p>導語:為了自己的暗戀事業和人生目標,陸漓拼盡一切抓住機會,終於和姜逸城達成一年的“契約夫婦”。兩人在同居中展開甜蜜互動,姜逸城對陸漓漸漸動心而不自知……</p>
</div>
</div>
</div>
</body>
</html>
CSS
body {
font-size: 1.125rem;
color: #707070;
margin: 0;
}
/* Typography */
h1 {
font-size: 3rem;
color: #212614;
text-align: center;
}
h1 span{
color: #b7832f;
}
h2 {
font-size: 1.5rem;
}
p {
margin-top: 0;
}
/* Layout */
img {
max-width: 100%;
}
.container {
width: 95%;
max-width: 980px;
margin: 0 auto;
}
.columns {
display: flex;
margin: 1em 0;
}
.col-1 {
width: 25%;
}
.col-2 {
width: 50%;
}
.col-3 {
width: 75%;
}
.col-bg {
background: #212614;
color: #fff;
padding: 1em;
}
15. Spacing out the columns
justify-content
Starting to think responsively
投影片範例
CSS
.parent {
display: flex;
justify-content: space-between;
}
- justify-content: space-between;
- justify-content: space-around;
- justify-content: space-evenly;
程式碼範例
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>intro to rwd</title>
<link rel="stylesheet" href="css/all.css">
</head>
<body>
<div class="container">
<h1>程序員那麼<span>可愛</span></h1>
<img src="images/banner.jpg" alt="banner jpg">
<div class="columns">
<div class="col col-1">
<h2>關於愛情 EP01</h2>
<p>暗戀就像是等待測試的程序,不確定運行結果,也不確定運行時間,但只要需求沒變,就要努力優化程序,直到它成功運行。</p>
</div>
<div class="col col-2">
<h2>關於愛情 EP02、EP03</h2>
<p>喜歡你這件事,不會有任何BUG,所以不需要DEBUG。</p>
<p>和暗戀的人在一起時,記得敲一遍高精度,這樣對他的喜歡,就不會溢出,被對方發現了。</p>
</div>
<div class="col col-1 col-bg">
<p>劇情簡介:陸漓(祝緒丹飾)是女程式設計師。為了追求理想而投身編程領域,她憑藉自身經歷及過人的智慧搞定學長姜逸城(邢昭林飾),擺平他無數次的相親後,成功進入異承公司,勇闖IT世界。倆人因程序代碼結緣,後在機緣巧合下成為同居的室友。性格迥異的他們在同居相處中鬥智鬥勇情況下,是否會意外觸發心動的代碼?</p>
</div>
</div>
<div class="columns">
<div class="col col-3">
<h2>關於愛情 EP04</h2>
<p>每個人都有屬於自己的秘密,雖然可以通過技術手段進行加密,但卻不知道系統何時會被黑客入侵,將我們保守的秘密公之於眾。</p>
</div>
<div class="col col-1 col-bg">
<p>導語:為了自己的暗戀事業和人生目標,陸漓拼盡一切抓住機會,終於和姜逸城達成一年的“契約夫婦”。兩人在同居中展開甜蜜互動,姜逸城對陸漓漸漸動心而不自知……</p>
</div>
</div>
</div>
</body>
</html>
CSS
body {
font-size: 1.125rem;
color: #707070;
margin: 0;
}
/* Typography */
h1 {
font-size: 3rem;
color: #212614;
text-align: center;
}
h1 span{
color: #b7832f;
}
h2 {
font-size: 1.5rem;
}
p {
margin-top: 0;
}
/* Layout */
img {
max-width: 100%;
}
.container {
width: 95%;
max-width: 980px;
margin: 0 auto;
}
.columns {
display: flex;
justify-content: space-between;
margin: 1em 0;
}
.col-1 {
width: 20%;
}
.col-2 {
width: 45%;
}
.col-3 {
width: 70%;
}
.col-bg {
background: #212614;
color: #fff;
padding: 1em;
}
16. Controlling the vertical position of flex items
algin-items
Starting to think responsively
投影片範例
CSS
.parent {
display: flex;
align-items: flex-start;
}
- align-items: flex-start;
- align-items: center;
- align-items: flex-end;
- align-items: stretch;
程式碼範例
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>intro to rwd</title>
<link rel="stylesheet" href="css/all.css">
</head>
<body>
<div class="container">
<h1>程序員那麼<span>可愛</span></h1>
<img src="images/banner.jpg" alt="banner jpg">
<div class="columns">
<div class="col col-1">
<h2>關於愛情 EP01</h2>
<p>暗戀就像是等待測試的程序,不確定運行結果,也不確定運行時間,但只要需求沒變,就要努力優化程序,直到它成功運行。</p>
</div>
<div class="col col-2">
<h2>關於愛情 EP02、EP03</h2>
<p>喜歡你這件事,不會有任何BUG,所以不需要DEBUG。</p>
<p>和暗戀的人在一起時,記得敲一遍高精度,這樣對他的喜歡,就不會溢出,被對方發現了。</p>
</div>
<div class="col col-1 col-bg">
<p>劇情簡介:陸漓(祝緒丹飾)是女程式設計師。為了追求理想而投身編程領域,她憑藉自身經歷及過人的智慧搞定學長姜逸城(邢昭林飾),擺平他無數次的相親後,成功進入異承公司,勇闖IT世界。倆人因程序代碼結緣,後在機緣巧合下成為同居的室友。性格迥異的他們在同居相處中鬥智鬥勇情況下,是否會意外觸發心動的代碼?</p>
</div>
</div>
<div class="columns">
<div class="col col-3">
<h2>關於愛情 EP04</h2>
<p>每個人都有屬於自己的秘密,雖然可以通過技術手段進行加密,但卻不知道系統何時會被黑客入侵,將我們保守的秘密公之於眾。</p>
</div>
<div class="col col-1 col-bg">
<p>導語:為了自己的暗戀事業和人生目標,陸漓拼盡一切抓住機會,終於和姜逸城達成一年的“契約夫婦”。兩人在同居中展開甜蜜互動,姜逸城對陸漓漸漸動心而不自知……</p>
</div>
</div>
</div>
</body>
</html>
CSS
body {
font-size: 1.125rem;
color: #707070;
margin: 0;
}
/* Typography */
h1 {
font-size: 3rem;
color: #212614;
text-align: center;
}
h1 span{
color: #b7832f;
}
h2 {
font-size: 1.5rem;
}
p {
margin-top: 0;
}
/* Layout */
img {
max-width: 100%;
}
.container {
width: 95%;
max-width: 980px;
margin: 0 auto;
}
.columns {
display: flex;
justify-content: space-between;
align-items: flex-start;
margin: 1em 0;
}
.col-1 {
width: 20%;
}
.col-2 {
width: 45%;
}
.col-3 {
width: 70%;
}
.col-bg {
background: #212614;
color: #fff;
padding: 1em;
}
17. Media Query basics
Media queries – the basics
Starting to think responsively
- Media queries let us add new styles that target only specific conditions
Media queries – basic syntax
@media () { … }
Media queries – basic syntax
@media media-type () { … }
Media queries – basic syntax
@media media-type and (media-features) { … }
Media queries – media type
The media type let’s us target different types of media
- Screen @media screen { … }
- Print @media print { … }
- Speech @media speech { … }
Media queries – media condition
The media condition let’s us target specific conditions within that media type
- Widths @media (min-width: 600px) { … }
- Orientation @media (orientation: landscape) { … }
- Specific features @media (hover) { … }
Media queries
Both media types and conditions are optional.
We do need to either have a type or condition though.
Media queries
For example, we can target only screens.
@media screen { … }
Media queries
Or we can choose only a condition, such as the width of the viewport.
@media (min-width: 960px) { … }
Media queries
You can combine a type with a condition by using and.
@media screen and (min-width: 960px) { … }
@media screen and (orientation: portrait) { … }
- For now, we’re focused on min-width and max-width
- Let’s start with a simple example
程式碼範例
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>intro to rwd</title>
<link rel="stylesheet" href="css/all.css">
</head>
<body>
<div class="container">
<h1>程序員那麼<span>可愛</span></h1>
<img src="images/banner.jpg" alt="banner jpg">
<div class="columns">
<div class="col col-1">
<h2>關於愛情 EP01</h2>
<p>暗戀就像是等待測試的程序,不確定運行結果,也不確定運行時間,但只要需求沒變,就要努力優化程序,直到它成功運行。</p>
</div>
<div class="col col-2">
<h2>關於愛情 EP02、EP03</h2>
<p>喜歡你這件事,不會有任何BUG,所以不需要DEBUG。</p>
<p>和暗戀的人在一起時,記得敲一遍高精度,這樣對他的喜歡,就不會溢出,被對方發現了。</p>
</div>
<div class="col col-1 col-bg">
<p>劇情簡介:陸漓(祝緒丹飾)是女程式設計師。為了追求理想而投身編程領域,她憑藉自身經歷及過人的智慧搞定學長姜逸城(邢昭林飾),擺平他無數次的相親後,成功進入異承公司,勇闖IT世界。倆人因程序代碼結緣,後在機緣巧合下成為同居的室友。性格迥異的他們在同居相處中鬥智鬥勇情況下,是否會意外觸發心動的代碼?</p>
</div>
</div>
<div class="columns">
<div class="col col-3">
<h2>關於愛情 EP04</h2>
<p>每個人都有屬於自己的秘密,雖然可以通過技術手段進行加密,但卻不知道系統何時會被黑客入侵,將我們保守的秘密公之於眾。</p>
</div>
<div class="col col-1 col-bg">
<p>導語:為了自己的暗戀事業和人生目標,陸漓拼盡一切抓住機會,終於和姜逸城達成一年的“契約夫婦”。兩人在同居中展開甜蜜互動,姜逸城對陸漓漸漸動心而不自知……</p>
</div>
</div>
</div>
</body>
</html>
CSS
body {
font-size: 1.125rem;
color: #707070;
margin: 0;
background: pink;
}
/* from a minimun width of 650px and bigger */
@media (min-width: 650px) {
body {
background: orange;
}
}
/* from a minimun width of 400px up to a width of 649px */
@media (min-width: 400px) and (max-width: 649px) {
body {
background: purple;
}
}
/* Typography */
h1 {
font-size: 3rem;
color: #212614;
text-align: center;
}
h1 span{
color: #b7832f;
}
h2 {
font-size: 1.5rem;
}
p {
margin-top: 0;
}
/* Layout */
img {
max-width: 100%;
}
.container {
width: 95%;
max-width: 980px;
margin: 0 auto;
}
.columns {
display: flex;
justify-content: space-between;
align-items: flex-start;
margin: 1em 0;
}
.col-1 {
width: 20%;
}
.col-2 {
width: 45%;
}
.col-3 {
width: 70%;
}
.col-bg {
background: #212614;
color: #fff;
padding: 1em;
}
18. Making out layout responsive with flex-direction
Making our layout fully responsive
Starting to think responsively
Flexbox – changing the axis around
投影片範例
display: flex /* creates columns */
flex-direction: row; /* default behavior */
Flexbox – changing the axis around
display: flex /* creates columns */
flex-direction: column; /* switches the axis */
.parent {
display: flex;
flex-direction: column;
}
- Let’s give it a try
程式碼範例 – 方式一
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>intro to rwd</title>
<link rel="stylesheet" href="css/all.css">
</head>
<body>
<div class="container">
<h1>程序員那麼<span>可愛</span></h1>
<img src="images/banner.jpg" alt="banner jpg">
<div class="columns">
<div class="col col-1">
<h2>關於愛情 EP01</h2>
<p>暗戀就像是等待測試的程序,不確定運行結果,也不確定運行時間,但只要需求沒變,就要努力優化程序,直到它成功運行。</p>
</div>
<div class="col col-2">
<h2>關於愛情 EP02、EP03</h2>
<p>喜歡你這件事,不會有任何BUG,所以不需要DEBUG。</p>
<p>和暗戀的人在一起時,記得敲一遍高精度,這樣對他的喜歡,就不會溢出,被對方發現了。</p>
</div>
<div class="col col-1 col-bg">
<p>劇情簡介:陸漓(祝緒丹飾)是女程式設計師。為了追求理想而投身編程領域,她憑藉自身經歷及過人的智慧搞定學長姜逸城(邢昭林飾),擺平他無數次的相親後,成功進入異承公司,勇闖IT世界。倆人因程序代碼結緣,後在機緣巧合下成為同居的室友。性格迥異的他們在同居相處中鬥智鬥勇情況下,是否會意外觸發心動的代碼?</p>
</div>
</div>
<div class="columns">
<div class="col col-3">
<h2>關於愛情 EP04</h2>
<p>每個人都有屬於自己的秘密,雖然可以通過技術手段進行加密,但卻不知道系統何時會被黑客入侵,將我們保守的秘密公之於眾。</p>
</div>
<div class="col col-1 col-bg">
<p>導語:為了自己的暗戀事業和人生目標,陸漓拼盡一切抓住機會,終於和姜逸城達成一年的“契約夫婦”。兩人在同居中展開甜蜜互動,姜逸城對陸漓漸漸動心而不自知……</p>
</div>
</div>
</div>
</body>
</html>
CSS – 方式一
body {
font-size: 1.125rem;
color: #707070;
margin: 0;
}
/* Typography */
h1 {
font-size: 3rem;
color: #212614;
text-align: center;
}
h1 span{
color: #b7832f;
}
h2 {
font-size: 1.5rem;
}
p {
margin-top: 0;
}
/* Layout */
img {
max-width: 100%;
}
.container {
width: 95%;
max-width: 980px;
margin: 0 auto;
}
.columns {
display: flex;
justify-content: space-between;
align-items: flex-start;
margin: 1em 0;
}
@media (max-width: 600px) {
.columns {
flex-direction: column;
}
}
.col-1 {
width: 20%;
}
.col-2 {
width: 45%;
}
.col-3 {
width: 70%;
}
@media (max-width: 600px) {
.col-1,
.col-2,
.col-3 {
width: 100%;
}
}
.col-bg {
background: #212614;
color: #fff;
padding: 1em;
}
CSS – 方式二
body {
font-size: 1.125rem;
color: #707070;
margin: 0;
}
/* Typography */
h1 {
font-size: 3rem;
color: #212614;
text-align: center;
}
h1 span{
color: #b7832f;
}
h2 {
font-size: 1.5rem;
}
p {
margin-top: 0;
}
/* Layout */
img {
max-width: 100%;
}
.container {
width: 95%;
max-width: 980px;
margin: 0 auto;
}
.columns {
display: flex;
justify-content: space-between;
align-items: flex-start;
margin: 1em 0;
}
.col-1 {
width: 20%;
}
.col-2 {
width: 45%;
}
.col-3 {
width: 70%;
}
.col-bg {
background: #212614;
color: #fff;
padding: 1em;
}
@media (max-width: 600px) {
.columns {
flex-direction: column;
}
.col-1,
.col-2,
.col-3 {
width: 100%;
}
}
19. flex-direction explained
Flex-direction explained
Starting to think responsively
Flexbox – changing the axis around
display: flex; /* creates columns */
flex-direction: row; /* default behavior */
Flexbox – changing the axis around
display: flex; /* creates columns */
flex-direction: column; /* switches the axis */
投影片範例
.parent {
display: flex;
flex-direction: column;
}
- We are switching the main axis
投影片範例
.parent {
display: flex;
justify-content: space-between;
}
投影片範例
.parent {
display: flex;
justify-content: space-between;
flex-direction: column;
}
- justify-content will now work vertically
align-items will now work horizontally
投影片範例
.parent {
display: flex;
align-items: flex-start;
}
.parent {
display: flex;
align-items: flex-start;
flex-direction: column;
}
投影片範例
.parent {
display: flex;
align-items: center;
}
.parent {
display: flex;
align-items: center;
flex-direction: column;
}
.parent {
display: flex;
flex-direction: column;
align-items: center;
justify-content: space-between;
}
20. Creating a navigation
Creating a navigation
Starting to think responsively
Creating a navigation
All this flex stuff really comes into play when styling a responsive navigation for a site.
Creating a navigation
Navigations are traditionally marked up using an unordered list, which is placed inside a <nav> element.
Creating a navigation
<nav>
<ul>
<li><a href="index.html">home</a></li>
<li><a href="about.html">about me</a></li>
<li><a href="recent-posts.html">recent posts</a></li>
<ul>
</nav>
Colors
- #f8f8f8
- #707070
- #1792d2
- #143774
font-sizes
- Title: Lora – 3.375rem
- Subtitle: Ubuntu bold – 1rem
- Navigation: Ubuntu bold – 1rem
程式碼範例
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>intro to rwd</title>
<link href="https://fonts.googleapis.com/css2?family=Lora&family=Ubuntu:wght@700&display=swap" rel="stylesheet">
<link rel="stylesheet" href="css/all.css">
</head>
<body>
<header>
<div class="site-title">
<h1>程序員那麼可愛</h1>
<p class="subtitle">Cute Programmer</p>
</div>
<nav>
<ul>
<li><a href="#">首頁</a></li>
<li><a href="#">關於愛情</a></li>
<li><a href="#">劇情介紹</a></li>
</ul>
</nav>
</header>
</body>
</html>
CSS
/* Fonts
-----
font-family: 'Lora', serif;
font-family: 'Ubuntu', sans-serif;
*/
21. Using flexbox to start styling our navigation
程式碼範例
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>intro to rwd</title>
<link href="https://fonts.googleapis.com/css2?family=Lora&family=Ubuntu:wght@700&display=swap" rel="stylesheet">
<link rel="stylesheet" href="css/all.css">
</head>
<body>
<header>
<div class="site-title">
<h1>程序員那麼可愛</h1>
<p class="subtitle">Cute Programmer</p>
</div>
<nav>
<ul>
<li><a href="#">首頁</a></li>
<li><a href="#">關於愛情</a></li>
<li><a href="#">劇情介紹</a></li>
</ul>
</nav>
</header>
</body>
</html>
CSS
/* Fonts
-----
font-family: 'Lora', serif;
font-family: 'Ubuntu', sans-serif;
*/
header {
text-align: center;
}
nav ul {
list-style: none;
padding: 0;
display: flex;
justify-content: center;
}
nav li {
margin: 0 1em;
}
nav a {
}
nav a:hover {
}
22. Making out navigation look good
程式碼範例
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>intro to rwd</title>
<link href="https://fonts.googleapis.com/css2?family=Lora&family=Ubuntu:wght@700&display=swap" rel="stylesheet">
<link rel="stylesheet" href="css/all.css">
</head>
<body>
<header>
<div class="site-title">
<h1>程序員那麼可愛</h1>
<p class="subtitle">Cute Programmer</p>
</div>
<nav>
<ul>
<li><a href="#">首頁</a></li>
<li><a href="#">關於愛情</a></li>
<li><a href="#">劇情介紹</a></li>
</ul>
</nav>
</header>
</body>
</html>
CSS
/* Fonts
-----
font-family: 'Lora', serif;
font-family: 'Ubuntu', sans-serif;
*/
body {
margin: 0;
font-family: 'Ubuntu', sans-serif;
}
/* =================
Typography
================= */
h1 {
font-family: 'Lora', serif;
font-weight: 400;
color: #143774;
font-size: 2rem;
margin: 0;
}
.subtitle {
font-weight: 700;
color: #1792d2;
font-size: 0.75rem;
margin: 0;
}
header {
text-align: center;
background: #f8f8f8;
padding: 2em 0;
}
nav ul {
list-style: none;
padding: 0;
display: flex;
justify-content: center;
}
nav li {
margin: 0 1em;
}
nav a {
text-decoration: none;
color: #707070;
font-weight: 700;
}
nav a:hover,
nav a:focus {
color: #1792d2;
}
23. Adding the underline
程式碼範例
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>intro to rwd</title>
<link href="https://fonts.googleapis.com/css2?family=Lora&family=Ubuntu:wght@700&display=swap" rel="stylesheet">
<link rel="stylesheet" href="css/all.css">
</head>
<body>
<header>
<div class="site-title">
<h1>程序員那麼可愛</h1>
<p class="subtitle">Cute Programmer</p>
</div>
<nav>
<ul>
<li><a href="#" class="current-page">首頁</a></li>
<li><a href="#">關於愛情</a></li>
<li><a href="#">劇情介紹</a></li>
</ul>
</nav>
</header>
</body>
</html>
CSS
/* Fonts
-----
font-family: 'Lora', serif;
font-family: 'Ubuntu', sans-serif;
*/
body {
margin: 0;
font-family: 'Ubuntu', sans-serif;
}
/* =================
Typography
================= */
h1 {
font-family: 'Lora', serif;
font-weight: 400;
color: #143774;
font-size: 2rem;
margin: 0;
}
.subtitle {
font-weight: 700;
color: #1792d2;
font-size: 0.75rem;
margin: 0;
}
/* =================
Layout
================= */
header {
text-align: center;
background: #f8f8f8;
padding: 2em 0;
}
/* navigation */
nav ul {
list-style: none;
padding: 0;
display: flex;
justify-content: center;
}
nav li {
margin: 0 1em;
}
nav a {
text-decoration: none;
color: #707070;
font-weight: 700;
padding: 0.25em 0;
}
nav a:hover,
nav a:focus {
color: #1792d2;
}
.current-page {
border-bottom: 1px solid #707070;
}
.current-page:hover {
color: #707070;
}
24. A more complicated navigation
程式碼範例
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>intro to rwd</title>
<link href="https://fonts.googleapis.com/css2?family=Lora&family=Ubuntu:wght@700&display=swap" rel="stylesheet">
<link rel="stylesheet" href="css/all.css">
</head>
<body>
<header>
<div class="container container-nav">
<div class="site-title">
<h1>程序員那麼可愛</h1>
<p class="subtitle">Cute Programmer</p>
</div>
<nav>
<ul>
<li><a href="#" class="current-page">首頁</a></li>
<li><a href="#">關於愛情</a></li>
<li><a href="#">劇情介紹</a></li>
</ul>
</nav>
</div> <!-- / .container -->
</header>
</body>
</html>
CSS
/* Fonts
-----
font-family: 'Lora', serif;
font-family: 'Ubuntu', sans-serif;
*/
body {
margin: 0;
font-family: 'Ubuntu', sans-serif;
}
/* =================
Typography
================= */
h1 {
font-family: 'Lora', serif;
font-weight: 400;
color: #143774;
font-size: 2rem;
margin: 0;
}
.subtitle {
font-weight: 700;
color: #1792d2;
font-size: 0.75rem;
margin: 0;
}
/* =================
Layout
================= */
.container {
width: 90%;
max-width: 900px;
margin: 0 auto;
}
.container-nav {
display: flex;
justify-content: space-between;
}
header {
background: #f8f8f8;
padding: 2em 0;
}
/* navigation */
nav ul {
list-style: none;
padding: 0;
display: flex;
justify-content: center;
}
nav li {
margin-left: 2em;
}
nav a {
text-decoration: none;
color: #707070;
font-weight: 700;
padding: 0.25em 0;
}
nav a:hover,
nav a:focus {
color: #1792d2;
}
.current-page {
border-bottom: 1px solid #707070;
}
.current-page:hover {
color: #707070;
}
25. Making the navigation responsive
Adding a media query to our nav
Starting to think responsively
程式碼範例
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>intro to rwd</title>
<link href="https://fonts.googleapis.com/css2?family=Lora&family=Ubuntu:wght@700&display=swap" rel="stylesheet">
<link rel="stylesheet" href="css/all.css">
</head>
<body>
<header>
<div class="container container-nav">
<div class="site-title">
<h1>程序員那麼可愛</h1>
<p class="subtitle">Cute Programmer</p>
</div>
<nav>
<ul>
<li><a href="#" class="current-page">首頁</a></li>
<li><a href="#">關於愛情</a></li>
<li><a href="#">劇情介紹</a></li>
</ul>
</nav>
</div> <!-- / .container -->
</header>
</body>
</html>
CSS
/* Fonts
-----
font-family: 'Lora', serif;
font-family: 'Ubuntu', sans-serif;
*/
body {
margin: 0;
font-family: 'Ubuntu', sans-serif;
}
/* =================
Typography
================= */
h1 {
font-family: 'Lora', serif;
font-weight: 400;
color: #143774;
font-size: 2rem;
margin: 0;
}
.subtitle {
font-weight: 700;
color: #1792d2;
font-size: 0.75rem;
margin: 0;
}
/* =================
Layout
================= */
.container {
width: 90%;
max-width: 900px;
margin: 0 auto;
}
.container-nav {
display: flex;
justify-content: space-between;
}
header {
background: #f8f8f8;
padding: 2em 0;
}
@media (max-width: 675px) {
.container-nav {
flex-direction: column;
}
header {
text-align: center;
}
}
/* navigation */
nav ul {
list-style: none;
padding: 0;
display: flex;
justify-content: center;
}
nav li {
margin-left: 2em;
}
nav a {
text-decoration: none;
color: #707070;
font-weight: 700;
padding: 0.25em 0;
}
nav a:hover,
nav a:focus {
color: #1792d2;
}
.current-page {
border-bottom: 1px solid #707070;
}
.current-page:hover {
color: #707070;
}
@media (max-width: 675px) {
nav ul {
flex-direction: column;
}
nav li {
margin: 0.5em 0;
}
}
26. Taking a look at the rest of the project
投影片範例 – 5 Screens
- simple life – mobile with navigation – 1
- simple life – mobile with navigation
- Simple life – with navigation
- Simple life about – with navigation
- Simple life recent posts – with navigation
資源
Adobe XD 設計稿文件講解
27. Setting up the structure
投影片講解 – HOME
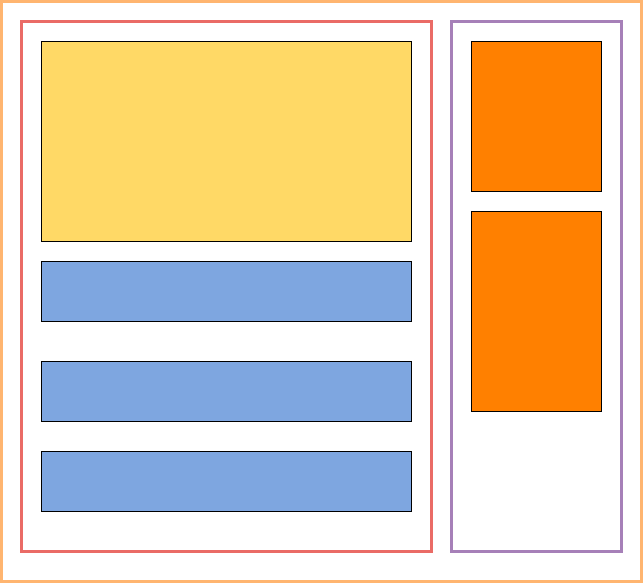
- Adobe XD 設計稿 – 連結
程式碼範例
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>intro to rwd</title>
<link href="https://fonts.googleapis.com/css2?family=Lora&family=Ubuntu:wght@700&display=swap" rel="stylesheet">
<link rel="stylesheet" href="css/all.css">
</head>
<body>
<header>
<div class="container container-nav">
<div class="site-title">
<h1>程序員那麼可愛</h1>
<p class="subtitle">Cute Programmer</p>
</div>
<nav>
<ul>
<li><a href="#" class="current-page">首頁</a></li>
<li><a href="#">關於愛情</a></li>
<li><a href="#">劇情介紹</a></li>
</ul>
</nav>
</div> <!-- / .container -->
</header>
<div class="container">
<main role="main"></main>
<aside class="sidebar"></aside>
</div>
<footer>
<p></p>
<p></p>
</footer>
</body>
</html>
CSS
/* Fonts
-----
font-family: 'Lora', serif;
font-family: 'Ubuntu', sans-serif;
*/
body {
margin: 0;
font-family: 'Ubuntu', sans-serif;
}
/* =================
Typography
================= */
h1 {
font-family: 'Lora', serif;
font-weight: 400;
color: #143774;
font-size: 2rem;
margin: 0;
}
.subtitle {
font-weight: 700;
color: #1792d2;
font-size: 0.75rem;
margin: 0;
}
/* =================
Layout
================= */
.container {
width: 90%;
max-width: 900px;
margin: 0 auto;
}
.container-nav {
display: flex;
justify-content: space-between;
}
header {
background: #f8f8f8;
padding: 2em 0;
}
@media (max-width: 675px) {
.container-nav {
flex-direction: column;
}
header {
text-align: center;
}
}
/* navigation */
nav ul {
list-style: none;
padding: 0;
display: flex;
justify-content: center;
}
nav li {
margin-left: 2em;
}
nav a {
text-decoration: none;
color: #707070;
font-weight: 700;
padding: 0.25em 0;
}
nav a:hover,
nav a:focus {
color: #1792d2;
}
.current-page {
border-bottom: 1px solid #707070;
}
.current-page:hover {
color: #707070;
}
@media (max-width: 675px) {
nav ul {
flex-direction: column;
}
nav li {
margin: 0.5em 0;
}
}
28. Featured article structure
程式碼範例
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>intro to rwd</title>
<link href="https://fonts.googleapis.com/css2?family=Lora&family=Ubuntu:wght@700&display=swap" rel="stylesheet">
<link rel="stylesheet" href="css/all.css">
</head>
<body>
<header>
<div class="container container-nav">
<div class="site-title">
<h1>程序員那麼可愛</h1>
<p class="subtitle">Cute Programmer</p>
</div>
<nav>
<ul>
<li><a href="#" class="current-page">首頁</a></li>
<li><a href="#">關於愛情</a></li>
<li><a href="#">劇情介紹</a></li>
</ul>
</nav>
</div> <!-- / .container -->
</header>
<div class="container">
<main role="main">
<article class="article-featured">
<h2 class="article-title"></h2>
<img src="" alt="" class="article-image">
<p class="article-info"></p>
<p class="article-body"></p>
<a href="#" class="article-read-more"></a>
</article>
<article class="article-recent"></article>
<article class="article-recent"></article>
<article class="article-recent"></article>
</main>
<aside class="sidebar"></aside>
</div>
<footer>
<p></p>
<p></p>
</footer>
</body>
</html>
CSS
/* Fonts
-----
font-family: 'Lora', serif;
font-family: 'Ubuntu', sans-serif;
*/
body {
margin: 0;
font-family: 'Ubuntu', sans-serif;
}
/* =================
Typography
================= */
h1 {
font-family: 'Lora', serif;
font-weight: 400;
color: #143774;
font-size: 2rem;
margin: 0;
}
.subtitle {
font-weight: 700;
color: #1792d2;
font-size: 0.75rem;
margin: 0;
}
/* =================
Layout
================= */
.container {
width: 90%;
max-width: 900px;
margin: 0 auto;
}
.container-nav {
display: flex;
justify-content: space-between;
}
header {
background: #f8f8f8;
padding: 2em 0;
}
@media (max-width: 675px) {
.container-nav {
flex-direction: column;
}
header {
text-align: center;
}
}
/* navigation */
nav ul {
list-style: none;
padding: 0;
display: flex;
justify-content: center;
}
nav li {
margin-left: 2em;
}
nav a {
text-decoration: none;
color: #707070;
font-weight: 700;
padding: 0.25em 0;
}
nav a:hover,
nav a:focus {
color: #1792d2;
}
.current-page {
border-bottom: 1px solid #707070;
}
.current-page:hover {
color: #707070;
}
@media (max-width: 675px) {
nav ul {
flex-direction: column;
}
nav li {
margin: 0.5em 0;
}
}
29. The home page – HTML for the recent articles
程式碼範例
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>intro to rwd</title>
<link href="https://fonts.googleapis.com/css2?family=Lora&family=Ubuntu:wght@700&display=swap" rel="stylesheet">
<link rel="stylesheet" href="css/all.css">
</head>
<body>
<header>
<div class="container container-nav">
<div class="site-title">
<h1>程序員那麼可愛</h1>
<p class="subtitle">Cute Programmer</p>
</div>
<nav>
<ul>
<li><a href="#" class="current-page">首頁</a></li>
<li><a href="#">關於愛情</a></li>
<li><a href="#">劇情介紹</a></li>
</ul>
</nav>
</div> <!-- / .container -->
</header>
<div class="container">
<main role="main">
<article class="article-featured">
<h2 class="article-title"></h2>
<img src="" alt="" class="article-image">
<p class="article-info"></p>
<p class="article-body"></p>
<a href="#" class="article-read-more"></a>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title"></h2>
<p class="article-body"></p>
<a href="#" class="article-read-more"></a>
</div>
<div class="article-recent-secondary">
<img src="" alt="" class="article-image">
<p class="article-info"></p>
</div>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title"></h2>
<p class="article-body"></p>
<a href="#" class="article-read-more"></a>
</div>
<div class="article-recent-secondary">
<img src="" alt="" class="article-image">
<p class="article-info"></p>
</div>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title"></h2>
<p class="article-body"></p>
<a href="#" class="article-read-more"></a>
</div>
<div class="article-recent-secondary">
<img src="" alt="" class="article-image">
<p class="article-info"></p>
</div>
</article>
</main>
<aside class="sidebar"></aside>
</div>
<footer>
<p></p>
<p></p>
</footer>
</body>
</html>
CSS
/* Fonts
-----
font-family: 'Lora', serif;
font-family: 'Ubuntu', sans-serif;
*/
body {
margin: 0;
font-family: 'Ubuntu', sans-serif;
}
/* =================
Typography
================= */
h1 {
font-family: 'Lora', serif;
font-weight: 400;
color: #143774;
font-size: 2rem;
margin: 0;
}
.subtitle {
font-weight: 700;
color: #1792d2;
font-size: 0.75rem;
margin: 0;
}
/* =================
Layout
================= */
.container {
width: 90%;
max-width: 900px;
margin: 0 auto;
}
.container-nav {
display: flex;
justify-content: space-between;
}
header {
background: #f8f8f8;
padding: 2em 0;
}
@media (max-width: 675px) {
.container-nav {
flex-direction: column;
}
header {
text-align: center;
}
}
/* navigation */
nav ul {
list-style: none;
padding: 0;
display: flex;
justify-content: center;
}
nav li {
margin-left: 2em;
}
nav a {
text-decoration: none;
color: #707070;
font-weight: 700;
padding: 0.25em 0;
}
nav a:hover,
nav a:focus {
color: #1792d2;
}
.current-page {
border-bottom: 1px solid #707070;
}
.current-page:hover {
color: #707070;
}
@media (max-width: 675px) {
nav ul {
flex-direction: column;
}
nav li {
margin: 0.5em 0;
}
}
30. Home Page – HTML for the aside
程式碼範例
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>intro to rwd</title>
<link href="https://fonts.googleapis.com/css2?family=Lora&family=Ubuntu:wght@700&display=swap" rel="stylesheet">
<link rel="stylesheet" href="css/all.css">
</head>
<body>
<header>
<div class="container container-nav">
<div class="site-title">
<h1>程序員那麼可愛</h1>
<p class="subtitle">Cute Programmer</p>
</div>
<nav>
<ul>
<li><a href="#" class="current-page">首頁</a></li>
<li><a href="#">關於愛情</a></li>
<li><a href="#">劇情介紹</a></li>
</ul>
</nav>
</div> <!-- / .container -->
</header>
<div class="container">
<main role="main">
<article class="article-featured">
<h2 class="article-title"></h2>
<img src="" alt="" class="article-image">
<p class="article-info"></p>
<p class="article-body"></p>
<a href="#" class="article-read-more"></a>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title"></h2>
<p class="article-body"></p>
<a href="#" class="article-read-more"></a>
</div>
<div class="article-recent-secondary">
<img src="" alt="" class="article-image">
<p class="article-info"></p>
</div>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title"></h2>
<p class="article-body"></p>
<a href="#" class="article-read-more"></a>
</div>
<div class="article-recent-secondary">
<img src="" alt="" class="article-image">
<p class="article-info"></p>
</div>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title"></h2>
<p class="article-body"></p>
<a href="#" class="article-read-more"></a>
</div>
<div class="article-recent-secondary">
<img src="" alt="" class="article-image">
<p class="article-info"></p>
</div>
</article>
</main>
<aside class="sidebar">
<div class="sidebar-widget">
<h2 class="widget-title"></h2>
<img src="" alt="" class="widget-image">
<p class="widget-body"></p>
</div>
<div class="sidebar-widget">
<h2 class="widget-title"></h2>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title"></h3>
<img src="" alt="" class="widget-image">
</div>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title"></h3>
<img src="" alt="" class="widget-image">
</div>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title"></h3>
<img src="" alt="" class="widget-image">
</div>
</div>
</aside>
</div>
<footer>
<p></p>
<p></p>
</footer>
</body>
</html>
CSS
/* Fonts
-----
font-family: 'Lora', serif;
font-family: 'Ubuntu', sans-serif;
*/
body {
margin: 0;
font-family: 'Ubuntu', sans-serif;
}
/* =================
Typography
================= */
h1 {
font-family: 'Lora', serif;
font-weight: 400;
color: #143774;
font-size: 2rem;
margin: 0;
}
.subtitle {
font-weight: 700;
color: #1792d2;
font-size: 0.75rem;
margin: 0;
}
/* =================
Layout
================= */
.container {
width: 90%;
max-width: 900px;
margin: 0 auto;
}
.container-nav {
display: flex;
justify-content: space-between;
}
header {
background: #f8f8f8;
padding: 2em 0;
}
@media (max-width: 675px) {
.container-nav {
flex-direction: column;
}
header {
text-align: center;
}
}
/* navigation */
nav ul {
list-style: none;
padding: 0;
display: flex;
justify-content: center;
}
nav li {
margin-left: 2em;
}
nav a {
text-decoration: none;
color: #707070;
font-weight: 700;
padding: 0.25em 0;
}
nav a:hover,
nav a:focus {
color: #1792d2;
}
.current-page {
border-bottom: 1px solid #707070;
}
.current-page:hover {
color: #707070;
}
@media (max-width: 675px) {
nav ul {
flex-direction: column;
}
nav li {
margin: 0.5em 0;
}
}
31. Starting the CSS for our page
- Adobe XD 設計稿 – 連結
Colors
- #707070
- #1792d2
- #143774
Font-sizes
- Lora 2rem – Living the simple life、#143774
- Lora 1.5rem – Heading 2 (blog post titles)、#143774
- Ubuntu bold 0.75rem – SUBHEADING、#1792d2
- Ubuntu light 1.125rem – body text、#707070
- Ubuntu bold 0.875rem – CONTINUE READING、#1792d2
- Ubuntu light 0.875rem – Dates、#707070
程式碼範例
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>intro to rwd</title>
<link href="https://fonts.googleapis.com/css2?family=Lora&family=Ubuntu:wght@300;400;700&display=swap" rel="stylesheet">
<link rel="stylesheet" href="css/all.css">
</head>
<body>
<header>
<div class="container container-nav">
<div class="site-title">
<h1>程序員那麼可愛</h1>
<p class="subtitle">Cute Programmer</p>
</div>
<nav>
<ul>
<li><a href="#" class="current-page">首頁</a></li>
<li><a href="#">關於愛情</a></li>
<li><a href="#">劇情介紹</a></li>
</ul>
</nav>
</div> <!-- / .container -->
</header>
<div class="container">
<main role="main">
<article class="article-featured">
<h2 class="article-title">劇情介紹</h2>
<img src="images/banner.jpg" alt="banner jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
<p class="article-body">陸漓(<a href="#">祝緒丹</a>飾)是女程式設計師。為了追求理想而投身編程領域,她憑藉自身經歷及過人的智慧搞定學長姜逸城(<a href="#">邢昭林</a>飾),擺平他無數次的相親後,成功進入異承公司,勇闖IT世界。倆人因程序代碼結緣,後在機緣巧合下成為同居的室友。性格迥異的他們在同居相處中鬥智鬥勇情況下,<strong>是否會意外觸發心動的代碼?</strong></p>
<a href="#" class="article-read-more">繼續閱讀</a>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">姜逸城 (邢昭林飾)</h2>
<p class="article-body">28歲,陸漓的學長。性格傲嬌又自戀的霸道總裁。聰明的他是個名副其實的毒舌。喜歡在完全不講道理的情況下講道理。雖然懶散,但他是信奉效率和結果至上主義者,對員工要求極高。後在機緣巧合下成為陸漓的同居室友。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/01.jpg" alt="01 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">陸漓 (祝緒丹飾)</h2>
<p class="article-body">22歲,可愛學霸。暗戀姜逸城,是他同科系的學妹,曾幫他擺平無數次的相親。為了接近他而決定當個女程式設計師,經歷過無數關卡,甚至還女扮男裝,努力進入不接受收女程式設計師的異承公司。後在機緣巧合下成為姜逸城的同居室友。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/02.jpg" alt="02 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">顧筱柒 (關芯飾)</h2>
<p class="article-body">別名小七。陸漓的頭號閨蜜,二次元服裝網店的老闆。熱情活潑、精力充沛。遇到帥哥時,立馬化身為撩漢魔女,但每次戀愛總莫名無疾而終。性格馬虎,常常鬧出笑話來。與顧默是青梅竹馬,乾親至交。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/03.jpg" alt="03 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
</main>
<aside class="sidebar">
<div class="sidebar-widget">
<h2 class="widget-title">導語</h2>
<img src="images/cute-programmer.jpg" alt="cute-programmer jpg" class="widget-image">
<p class="widget-body">
為了自己的暗戀事業和人生目標,陸漓拼盡一切抓住機會,終於和姜逸城達成一年的“契約夫婦”。兩人在同居中展開甜蜜互動,姜逸城對陸漓漸漸動心而不自知……
</p>
</div>
<div class="sidebar-widget">
<h2 class="widget-title">最近文章</h2>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">姜逸城 (邢昭林飾)</h3>
<img src="images/01.jpg" alt="01 jpg" class="widget-image">
</div>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">陸漓 (祝緒丹飾)</h3>
<img src="images/02.jpg" alt="02 jpg" class="widget-image">
</div>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">顧筱柒 (關芯飾)</h3>
<img src="images/03.jpg" alt="03 jpg" class="widget-image">
</div>
</div>
</aside>
</div>
<footer>
<p></p>
<p></p>
</footer>
</body>
</html>
CSS
/* Fonts
-----
font-family: 'Lora', serif;
font-family: 'Ubuntu', sans-serif;
*/
body {
margin: 0;
font-family: 'Ubuntu', sans-serif;
font-size: 1.125rem;
font-weight: 300;
}
/* =================
Typography
================= */
h1,
h2,
h3 {
font-family: 'Lora', serif;
font-weight: 400;
color: #143774;
}
h1 {
font-size: 2rem;
margin: 0;
}
a {
color: #1792d2;
}
a:hover,
a:focus {
color: #143774;
}
strong {
font-weight: 700;
}
/* h1 subtitle */
.subtitle {
font-weight: 700;
color: #1792d2;
font-size: 0.75rem;
margin: 0;
}
.article-title {
font-size: 1.5rem;
}
.article-read-more,
.article-info {
font-size: 0.875rem;
}
.article-read-more {
color: #1792d2;
text-decoration: none;
font-weight: 700;
}
.article-read-more:hover,
.article-read-more:focus {
color: #143774;
text-decoration: underline;
}
/* =================
Layout
================= */
.container {
width: 90%;
max-width: 900px;
margin: 0 auto;
}
.container-nav {
display: flex;
justify-content: space-between;
}
header {
background: #f8f8f8;
padding: 2em 0;
}
@media (max-width: 675px) {
.container-nav {
flex-direction: column;
}
header {
text-align: center;
}
}
/* navigation */
nav ul {
list-style: none;
padding: 0;
display: flex;
justify-content: center;
}
nav li {
margin-left: 2em;
}
nav a {
text-decoration: none;
color: #707070;
font-weight: 700;
padding: 0.25em 0;
}
nav a:hover,
nav a:focus {
color: #1792d2;
}
.current-page {
border-bottom: 1px solid #707070;
}
.current-page:hover {
color: #707070;
}
@media (max-width: 675px) {
nav ul {
flex-direction: column;
}
nav li {
margin: 0.5em 0;
}
}
32. Starting the layout – looking at the big picture
程式碼範例
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>intro to rwd</title>
<link href="https://fonts.googleapis.com/css2?family=Lora&family=Ubuntu:wght@300;400;700&display=swap" rel="stylesheet">
<link rel="stylesheet" href="css/all.css">
</head>
<body>
<header>
<div class="container container-flex">
<div class="site-title">
<h1>程序員那麼可愛</h1>
<p class="subtitle">Cute Programmer</p>
</div>
<nav>
<ul>
<li><a href="#" class="current-page">首頁</a></li>
<li><a href="#">關於愛情</a></li>
<li><a href="#">劇情介紹</a></li>
</ul>
</nav>
</div> <!-- / .container -->
</header>
<div class="container container-flex">
<main role="main">
<article class="article-featured">
<h2 class="article-title">劇情介紹</h2>
<img src="images/banner.jpg" alt="banner jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
<p class="article-body">陸漓(<a href="#">祝緒丹</a>飾)是女程式設計師。為了追求理想而投身編程領域,她憑藉自身經歷及過人的智慧搞定學長姜逸城(<a href="#">邢昭林</a>飾),擺平他無數次的相親後,成功進入異承公司,勇闖IT世界。倆人因程序代碼結緣,後在機緣巧合下成為同居的室友。性格迥異的他們在同居相處中鬥智鬥勇情況下,<strong>是否會意外觸發心動的代碼?</strong></p>
<a href="#" class="article-read-more">繼續閱讀</a>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">姜逸城 (邢昭林飾)</h2>
<p class="article-body">28歲,陸漓的學長。性格傲嬌又自戀的霸道總裁。聰明的他是個名副其實的毒舌。喜歡在完全不講道理的情況下講道理。雖然懶散,但他是信奉效率和結果至上主義者,對員工要求極高。後在機緣巧合下成為陸漓的同居室友。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/01.jpg" alt="01 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">陸漓 (祝緒丹飾)</h2>
<p class="article-body">22歲,可愛學霸。暗戀姜逸城,是他同科系的學妹,曾幫他擺平無數次的相親。為了接近他而決定當個女程式設計師,經歷過無數關卡,甚至還女扮男裝,努力進入不接受收女程式設計師的異承公司。後在機緣巧合下成為姜逸城的同居室友。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/02.jpg" alt="02 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">顧筱柒 (關芯飾)</h2>
<p class="article-body">別名小七。陸漓的頭號閨蜜,二次元服裝網店的老闆。熱情活潑、精力充沛。遇到帥哥時,立馬化身為撩漢魔女,但每次戀愛總莫名無疾而終。性格馬虎,常常鬧出笑話來。與顧默是青梅竹馬,乾親至交。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/03.jpg" alt="03 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
</main>
<aside class="sidebar">
<div class="sidebar-widget">
<h2 class="widget-title">導語</h2>
<img src="images/cute-programmer.jpg" alt="cute-programmer jpg" class="widget-image">
<p class="widget-body">
為了自己的暗戀事業和人生目標,陸漓拼盡一切抓住機會,終於和姜逸城達成一年的“契約夫婦”。兩人在同居中展開甜蜜互動,姜逸城對陸漓漸漸動心而不自知……
</p>
</div>
<div class="sidebar-widget">
<h2 class="widget-title">最近文章</h2>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">姜逸城 (邢昭林飾)</h3>
<img src="images/01.jpg" alt="01 jpg" class="widget-image">
</div>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">陸漓 (祝緒丹飾)</h3>
<img src="images/02.jpg" alt="02 jpg" class="widget-image">
</div>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">顧筱柒 (關芯飾)</h3>
<img src="images/03.jpg" alt="03 jpg" class="widget-image">
</div>
</div>
</aside>
</div>
<footer>
<p></p>
<p></p>
</footer>
</body>
</html>
CSS
/* Fonts
-----
font-family: 'Lora', serif;
font-family: 'Ubuntu', sans-serif;
*/
body {
margin: 0;
font-family: 'Ubuntu', sans-serif;
font-size: 1.125rem;
font-weight: 300;
}
img {
max-width: 100%;
display: block;
}
/* =================
Typography
================= */
h1,
h2,
h3 {
font-family: 'Lora', serif;
font-weight: 400;
color: #143774;
}
h1 {
font-size: 2rem;
margin: 0;
}
a {
color: #1792d2;
}
a:hover,
a:focus {
color: #143774;
}
strong {
font-weight: 700;
}
/* h1 subtitle */
.subtitle {
font-weight: 700;
color: #1792d2;
font-size: 0.75rem;
margin: 0;
}
.article-title {
font-size: 1.5rem;
}
.article-read-more,
.article-info {
font-size: 0.875rem;
}
.article-read-more {
color: #1792d2;
text-decoration: none;
font-weight: 700;
}
.article-read-more:hover,
.article-read-more:focus {
color: #143774;
text-decoration: underline;
}
/* =================
Layout
================= */
.container {
width: 90%;
max-width: 900px;
margin: 0 auto;
}
.container-flex {
display: flex;
justify-content: space-between;
}
header {
background: #f8f8f8;
padding: 2em 0;
}
@media (max-width: 675px) {
.container-flex {
flex-direction: column;
}
header {
text-align: center;
}
}
main {
width: 75%;
}
aside {
width: 20%;
}
/* navigation */
nav ul {
list-style: none;
padding: 0;
display: flex;
justify-content: center;
}
nav li {
margin-left: 2em;
}
nav a {
text-decoration: none;
color: #707070;
font-weight: 700;
padding: 0.25em 0;
}
nav a:hover,
nav a:focus {
color: #1792d2;
}
.current-page {
border-bottom: 1px solid #707070;
}
.current-page:hover {
color: #707070;
}
@media (max-width: 675px) {
nav ul {
flex-direction: column;
}
nav li {
margin: 0.5em 0;
}
}
33. Starting to think mobile first
程式碼範例
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>intro to rwd</title>
<link href="https://fonts.googleapis.com/css2?family=Lora&family=Ubuntu:wght@300;400;700&display=swap" rel="stylesheet">
<link rel="stylesheet" href="css/all.css">
</head>
<body>
<header>
<div class="container container-flex">
<div class="site-title">
<h1>程序員那麼可愛</h1>
<p class="subtitle">Cute Programmer</p>
</div>
<nav>
<ul>
<li><a href="#" class="current-page">首頁</a></li>
<li><a href="#">關於愛情</a></li>
<li><a href="#">劇情介紹</a></li>
</ul>
</nav>
</div> <!-- / .container -->
</header>
<div class="container container-flex">
<main role="main">
<article class="article-featured">
<h2 class="article-title">劇情介紹</h2>
<img src="images/banner.jpg" alt="banner jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
<p class="article-body">陸漓(<a href="#">祝緒丹</a>飾)是女程式設計師。為了追求理想而投身編程領域,她憑藉自身經歷及過人的智慧搞定學長姜逸城(<a href="#">邢昭林</a>飾),擺平他無數次的相親後,成功進入異承公司,勇闖IT世界。倆人因程序代碼結緣,後在機緣巧合下成為同居的室友。性格迥異的他們在同居相處中鬥智鬥勇情況下,<strong>是否會意外觸發心動的代碼?</strong></p>
<a href="#" class="article-read-more">繼續閱讀</a>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">姜逸城 (邢昭林飾)</h2>
<p class="article-body">28歲,陸漓的學長。性格傲嬌又自戀的霸道總裁。聰明的他是個名副其實的毒舌。喜歡在完全不講道理的情況下講道理。雖然懶散,但他是信奉效率和結果至上主義者,對員工要求極高。後在機緣巧合下成為陸漓的同居室友。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/01.jpg" alt="01 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">陸漓 (祝緒丹飾)</h2>
<p class="article-body">22歲,可愛學霸。暗戀姜逸城,是他同科系的學妹,曾幫他擺平無數次的相親。為了接近他而決定當個女程式設計師,經歷過無數關卡,甚至還女扮男裝,努力進入不接受收女程式設計師的異承公司。後在機緣巧合下成為姜逸城的同居室友。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/02.jpg" alt="02 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">顧筱柒 (關芯飾)</h2>
<p class="article-body">別名小七。陸漓的頭號閨蜜,二次元服裝網店的老闆。熱情活潑、精力充沛。遇到帥哥時,立馬化身為撩漢魔女,但每次戀愛總莫名無疾而終。性格馬虎,常常鬧出笑話來。與顧默是青梅竹馬,乾親至交。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/03.jpg" alt="03 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
</main>
<aside class="sidebar">
<div class="sidebar-widget">
<h2 class="widget-title">導語</h2>
<img src="images/aboutme.jpg" alt="aboutme jpg" class="widget-image">
<p class="widget-body">
為了自己的暗戀事業和人生目標,陸漓拼盡一切抓住機會,終於和姜逸城達成一年的“契約夫婦”。兩人在同居中展開甜蜜互動,姜逸城對陸漓漸漸動心而不自知……
</p>
</div>
<div class="sidebar-widget">
<h2 class="widget-title">最近文章</h2>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">姜逸城 (邢昭林飾)</h3>
<img src="images/01.jpg" alt="01 jpg" class="widget-image">
</div>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">陸漓 (祝緒丹飾)</h3>
<img src="images/02.jpg" alt="02 jpg" class="widget-image">
</div>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">顧筱柒 (關芯飾)</h3>
<img src="images/03.jpg" alt="03 jpg" class="widget-image">
</div>
</div>
</aside>
</div>
<footer>
<p></p>
<p></p>
</footer>
</body>
</html>
CSS
/* Fonts
-----
font-family: 'Lora', serif;
font-family: 'Ubuntu', sans-serif;
*/
body {
margin: 0;
font-family: 'Ubuntu', sans-serif;
font-size: 1.125rem;
font-weight: 300;
}
img {
max-width: 100%;
display: block;
}
/* =================
Typography
================= */
h1,
h2,
h3 {
font-family: 'Lora', serif;
font-weight: 400;
color: #143774;
}
h1 {
font-size: 2rem;
margin: 0;
}
a {
color: #1792d2;
}
a:hover,
a:focus {
color: #143774;
}
strong {
font-weight: 700;
}
/* h1 subtitle */
.subtitle {
font-weight: 700;
color: #1792d2;
font-size: 0.75rem;
margin: 0;
}
.article-title {
font-size: 1.5rem;
}
.article-read-more,
.article-info {
font-size: 0.875rem;
}
.article-read-more {
color: #1792d2;
text-decoration: none;
font-weight: 700;
}
.article-read-more:hover,
.article-read-more:focus {
color: #143774;
text-decoration: underline;
}
/* =================
Layout
================= */
.container {
width: 90%;
max-width: 900px;
margin: 0 auto;
}
.container-flex {
display: flex;
flex-direction: column;
justify-content: space-between;
}
header {
background: #f8f8f8;
padding: 2em 0;
text-align: center;
}
@media (min-width: 675px) {
.container-flex {
flex-direction: row;
}
main {
width: 75%;
}
aside {
width: 20%;
}
}
/* navigation */
nav ul {
list-style: none;
padding: 0;
display: flex;
justify-content: center;
}
nav li {
margin-left: 2em;
}
nav a {
text-decoration: none;
color: #707070;
font-weight: 700;
padding: 0.25em 0;
}
nav a:hover,
nav a:focus {
color: #1792d2;
}
.current-page {
border-bottom: 1px solid #707070;
}
.current-page:hover {
color: #707070;
}
@media (max-width: 675px) {
nav ul {
flex-direction: column;
}
nav li {
margin: 0.5em 0;
}
}
34. Styling the featured article
程式碼範例
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>intro to rwd</title>
<link href="https://fonts.googleapis.com/css2?family=Lora&family=Ubuntu:wght@300;400;700&display=swap" rel="stylesheet">
<link rel="stylesheet" href="css/all.css">
</head>
<body>
<header>
<div class="container container-flex">
<div class="site-title">
<h1>程序員那麼可愛</h1>
<p class="subtitle">Cute Programmer</p>
</div>
<nav>
<ul>
<li><a href="#" class="current-page">首頁</a></li>
<li><a href="#">關於愛情</a></li>
<li><a href="#">劇情介紹</a></li>
</ul>
</nav>
</div> <!-- / .container -->
</header>
<div class="container container-flex">
<main role="main">
<article class="article-featured">
<h2 class="article-title">劇情介紹</h2>
<img src="images/banner.jpg" alt="banner jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
<p class="article-body">陸漓(<a href="#">祝緒丹</a>飾)是女程式設計師。為了追求理想而投身編程領域,她憑藉自身經歷及過人的智慧搞定學長姜逸城(<a href="#">邢昭林</a>飾),擺平他無數次的相親後,成功進入異承公司,勇闖IT世界。倆人因程序代碼結緣,後在機緣巧合下成為同居的室友。性格迥異的他們在同居相處中鬥智鬥勇情況下,<strong>是否會意外觸發心動的代碼?</strong></p>
<a href="#" class="article-read-more">繼續閱讀</a>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">姜逸城 (邢昭林飾)</h2>
<p class="article-body">28歲,陸漓的學長。性格傲嬌又自戀的霸道總裁。聰明的他是個名副其實的毒舌。喜歡在完全不講道理的情況下講道理。雖然懶散,但他是信奉效率和結果至上主義者,對員工要求極高。後在機緣巧合下成為陸漓的同居室友。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/01.jpg" alt="01 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">陸漓 (祝緒丹飾)</h2>
<p class="article-body">22歲,可愛學霸。暗戀姜逸城,是他同科系的學妹,曾幫他擺平無數次的相親。為了接近他而決定當個女程式設計師,經歷過無數關卡,甚至還女扮男裝,努力進入不接受收女程式設計師的異承公司。後在機緣巧合下成為姜逸城的同居室友。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/02.jpg" alt="02 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">顧筱柒 (關芯飾)</h2>
<p class="article-body">別名小七。陸漓的頭號閨蜜,二次元服裝網店的老闆。熱情活潑、精力充沛。遇到帥哥時,立馬化身為撩漢魔女,但每次戀愛總莫名無疾而終。性格馬虎,常常鬧出笑話來。與顧默是青梅竹馬,乾親至交。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/03.jpg" alt="03 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
</main>
<aside class="sidebar">
<div class="sidebar-widget">
<h2 class="widget-title">導語</h2>
<img src="images/aboutme.jpg" alt="aboutme jpg" class="widget-image">
<p class="widget-body">
為了自己的暗戀事業和人生目標,陸漓拼盡一切抓住機會,終於和姜逸城達成一年的“契約夫婦”。兩人在同居中展開甜蜜互動,姜逸城對陸漓漸漸動心而不自知……
</p>
</div>
<div class="sidebar-widget">
<h2 class="widget-title">最近文章</h2>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">姜逸城 (邢昭林飾)</h3>
<img src="images/01.jpg" alt="01 jpg" class="widget-image">
</div>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">陸漓 (祝緒丹飾)</h3>
<img src="images/02.jpg" alt="02 jpg" class="widget-image">
</div>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">顧筱柒 (關芯飾)</h3>
<img src="images/03.jpg" alt="03 jpg" class="widget-image">
</div>
</div>
</aside>
</div>
<footer>
<p></p>
<p></p>
</footer>
</body>
</html>
CSS
/* Fonts
-----
font-family: 'Lora', serif;
font-family: 'Ubuntu', sans-serif;
*/
body {
margin: 0;
font-family: 'Ubuntu', sans-serif;
font-size: 1.125rem;
font-weight: 300;
}
img {
max-width: 100%;
display: block;
}
/* =================
Typography
================= */
h1,
h2,
h3 {
font-family: 'Lora', serif;
font-weight: 400;
color: #143774;
}
h1 {
font-size: 2rem;
margin: 0;
}
a {
color: #1792d2;
}
a:hover,
a:focus {
color: #143774;
}
strong {
font-weight: 700;
}
/* h1 subtitle */
.subtitle {
font-weight: 700;
color: #1792d2;
font-size: 0.75rem;
margin: 0;
}
.article-title {
font-size: 1.5rem;
}
.article-read-more,
.article-info {
font-size: 0.875rem;
}
.article-read-more {
color: #1792d2;
text-decoration: none;
font-weight: 700;
}
.article-read-more:hover,
.article-read-more:focus {
color: #143774;
text-decoration: underline;
}
.article-info {
margin: 2em 0;
}
/* =================
Layout
================= */
.container {
width: 90%;
max-width: 900px;
margin: 0 auto;
}
.container-flex {
display: flex;
flex-direction: column;
justify-content: space-between;
}
header {
background: #f8f8f8;
padding: 2em 0;
text-align: center;
}
@media (min-width: 675px) {
.container-flex {
flex-direction: row;
}
main {
width: 75%;
}
aside {
width: 20%;
}
}
/* navigation */
nav ul {
list-style: none;
padding: 0;
display: flex;
justify-content: center;
}
nav li {
margin-left: 2em;
}
nav a {
text-decoration: none;
color: #707070;
font-weight: 700;
padding: 0.25em 0;
}
nav a:hover,
nav a:focus {
color: #1792d2;
}
.current-page {
border-bottom: 1px solid #707070;
}
.current-page:hover {
color: #707070;
}
@media (max-width: 675px) {
nav ul {
flex-direction: column;
}
nav li {
margin: 0.5em 0;
}
}
/* articles */
.article-featured {
border-bottom: 1px solid #707070;
padding-bottom: 2em;
margin-bottom: 2em;
}
35. Changing the visual order width flex box
程式碼範例
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>intro to rwd</title>
<link href="https://fonts.googleapis.com/css2?family=Lora&family=Ubuntu:wght@300;400;700&display=swap" rel="stylesheet">
<link rel="stylesheet" href="css/all.css">
</head>
<body>
<header>
<div class="container container-flex">
<div class="site-title">
<h1>程序員那麼可愛</h1>
<p class="subtitle">Cute Programmer</p>
</div>
<nav>
<ul>
<li><a href="#" class="current-page">首頁</a></li>
<li><a href="#">關於愛情</a></li>
<li><a href="#">劇情介紹</a></li>
</ul>
</nav>
</div> <!-- / .container -->
</header>
<div class="container container-flex">
<main role="main">
<article class="article-featured">
<h2 class="article-title">劇情介紹</h2>
<img src="images/banner.jpg" alt="banner jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
<p class="article-body">陸漓(<a href="#">祝緒丹</a>飾)是女程式設計師。為了追求理想而投身編程領域,她憑藉自身經歷及過人的智慧搞定學長姜逸城(<a href="#">邢昭林</a>飾),擺平他無數次的相親後,成功進入異承公司,勇闖IT世界。倆人因程序代碼結緣,後在機緣巧合下成為同居的室友。性格迥異的他們在同居相處中鬥智鬥勇情況下,<strong>是否會意外觸發心動的代碼?</strong></p>
<a href="#" class="article-read-more">繼續閱讀</a>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">姜逸城 (邢昭林飾)</h2>
<p class="article-body">28歲,陸漓的學長。性格傲嬌又自戀的霸道總裁。聰明的他是個名副其實的毒舌。喜歡在完全不講道理的情況下講道理。雖然懶散,但他是信奉效率和結果至上主義者,對員工要求極高。後在機緣巧合下成為陸漓的同居室友。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/01.jpg" alt="01 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">陸漓 (祝緒丹飾)</h2>
<p class="article-body">22歲,可愛學霸。暗戀姜逸城,是他同科系的學妹,曾幫他擺平無數次的相親。為了接近他而決定當個女程式設計師,經歷過無數關卡,甚至還女扮男裝,努力進入不接受收女程式設計師的異承公司。後在機緣巧合下成為姜逸城的同居室友。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/02.jpg" alt="02 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">顧筱柒 (關芯飾)</h2>
<p class="article-body">別名小七。陸漓的頭號閨蜜,二次元服裝網店的老闆。熱情活潑、精力充沛。遇到帥哥時,立馬化身為撩漢魔女,但每次戀愛總莫名無疾而終。性格馬虎,常常鬧出笑話來。與顧默是青梅竹馬,乾親至交。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/03.jpg" alt="03 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
</main>
<aside class="sidebar">
<div class="sidebar-widget">
<h2 class="widget-title">導語</h2>
<img src="images/aboutme.jpg" alt="aboutme jpg" class="widget-image">
<p class="widget-body">
為了自己的暗戀事業和人生目標,陸漓拼盡一切抓住機會,終於和姜逸城達成一年的“契約夫婦”。兩人在同居中展開甜蜜互動,姜逸城對陸漓漸漸動心而不自知……
</p>
</div>
<div class="sidebar-widget">
<h2 class="widget-title">最近文章</h2>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">姜逸城 (邢昭林飾)</h3>
<img src="images/01.jpg" alt="01 jpg" class="widget-image">
</div>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">陸漓 (祝緒丹飾)</h3>
<img src="images/02.jpg" alt="02 jpg" class="widget-image">
</div>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">顧筱柒 (關芯飾)</h3>
<img src="images/03.jpg" alt="03 jpg" class="widget-image">
</div>
</div>
</aside>
</div>
<footer>
<p></p>
<p></p>
</footer>
</body>
</html>
CSS
/* Fonts
-----
font-family: 'Lora', serif;
font-family: 'Ubuntu', sans-serif;
*/
body {
margin: 0;
font-family: 'Ubuntu', sans-serif;
font-size: 1.125rem;
font-weight: 300;
}
img {
max-width: 100%;
display: block;
}
/* =================
Typography
================= */
h1,
h2,
h3 {
font-family: 'Lora', serif;
font-weight: 400;
color: #143774;
margin-top: 0;
}
h1 {
font-size: 2rem;
margin: 0;
}
a {
color: #1792d2;
}
a:hover,
a:focus {
color: #143774;
}
strong {
font-weight: 700;
}
/* h1 subtitle */
.subtitle {
font-weight: 700;
color: #1792d2;
font-size: 0.75rem;
margin: 0;
}
.article-title {
font-size: 1.5rem;
}
.article-read-more,
.article-info {
font-size: 0.875rem;
}
.article-read-more {
color: #1792d2;
text-decoration: none;
font-weight: 700;
}
.article-read-more:hover,
.article-read-more:focus {
color: #143774;
text-decoration: underline;
}
.article-info {
margin: 2em 0;
}
/* =================
Layout
================= */
.container {
width: 90%;
max-width: 900px;
margin: 0 auto;
}
.container-flex {
display: flex;
flex-direction: column;
justify-content: space-between;
}
header {
background: #f8f8f8;
padding: 2em 0;
text-align: center;
}
@media (min-width: 675px) {
.container-flex {
flex-direction: row;
}
main {
width: 75%;
}
aside {
width: 20%;
}
}
/* navigation */
nav ul {
list-style: none;
padding: 0;
display: flex;
justify-content: center;
}
nav li {
margin-left: 2em;
}
nav a {
text-decoration: none;
color: #707070;
font-weight: 700;
padding: 0.25em 0;
}
nav a:hover,
nav a:focus {
color: #1792d2;
}
.current-page {
border-bottom: 1px solid #707070;
}
.current-page:hover {
color: #707070;
}
@media (max-width: 675px) {
nav ul {
flex-direction: column;
}
nav li {
margin: 0.5em 0;
}
}
/* articles */
.article-featured {
border-bottom: 1px solid #707070;
padding-bottom: 2em;
margin-bottom: 2em;
}
.article-recent {
display: flex;
flex-direction: column;
margin-bottom: 2em;
}
.article-recent-main {
order: 2;
}
.article-recent-secondary {
order: 1;
}
36. Playing with the title’s position, and the downsides of negative margins
程式碼範例
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>intro to rwd</title>
<link href="https://fonts.googleapis.com/css2?family=Lora&family=Ubuntu:wght@300;400;700&display=swap" rel="stylesheet">
<link rel="stylesheet" href="css/all.css">
</head>
<body>
<header>
<div class="container container-flex">
<div class="site-title">
<h1>程序員那麼可愛</h1>
<p class="subtitle">Cute Programmer</p>
</div>
<nav>
<ul>
<li><a href="#" class="current-page">首頁</a></li>
<li><a href="#">關於愛情</a></li>
<li><a href="#">劇情介紹</a></li>
</ul>
</nav>
</div> <!-- / .container -->
</header>
<div class="container container-flex">
<main role="main">
<article class="article-featured">
<h2 class="article-title">劇情介紹</h2>
<img src="images/banner.jpg" alt="banner jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
<p class="article-body">陸漓(<a href="#">祝緒丹</a>飾)是女程式設計師。為了追求理想而投身編程領域,她憑藉自身經歷及過人的智慧搞定學長姜逸城(<a href="#">邢昭林</a>飾),擺平他無數次的相親後,成功進入異承公司,勇闖IT世界。倆人因程序代碼結緣,後在機緣巧合下成為同居的室友。性格迥異的他們在同居相處中鬥智鬥勇情況下,<strong>是否會意外觸發心動的代碼?</strong></p>
<a href="#" class="article-read-more">繼續閱讀</a>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">姜逸城 (邢昭林飾)</h2>
<p class="article-body">28歲,陸漓的學長。性格傲嬌又自戀的霸道總裁。聰明的他是個名副其實的毒舌。喜歡在完全不講道理的情況下講道理。雖然懶散,但他是信奉效率和結果至上主義者,對員工要求極高。後在機緣巧合下成為陸漓的同居室友。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/01.jpg" alt="01 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">陸漓 (祝緒丹飾)</h2>
<p class="article-body">22歲,可愛學霸。暗戀姜逸城,是他同科系的學妹,曾幫他擺平無數次的相親。為了接近他而決定當個女程式設計師,經歷過無數關卡,甚至還女扮男裝,努力進入不接受收女程式設計師的異承公司。後在機緣巧合下成為姜逸城的同居室友。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/02.jpg" alt="02 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">顧筱柒 (關芯飾)</h2>
<p class="article-body">別名小七。陸漓的頭號閨蜜,二次元服裝網店的老闆。熱情活潑、精力充沛。遇到帥哥時,立馬化身為撩漢魔女,但每次戀愛總莫名無疾而終。性格馬虎,常常鬧出笑話來。與顧默是青梅竹馬,乾親至交。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/03.jpg" alt="03 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
</main>
<aside class="sidebar">
<div class="sidebar-widget">
<h2 class="widget-title">導語</h2>
<img src="images/aboutme.jpg" alt="aboutme jpg" class="widget-image">
<p class="widget-body">
為了自己的暗戀事業和人生目標,陸漓拼盡一切抓住機會,終於和姜逸城達成一年的“契約夫婦”。兩人在同居中展開甜蜜互動,姜逸城對陸漓漸漸動心而不自知……
</p>
</div>
<div class="sidebar-widget">
<h2 class="widget-title">最近文章</h2>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">姜逸城 (邢昭林飾)</h3>
<img src="images/01.jpg" alt="01 jpg" class="widget-image">
</div>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">陸漓 (祝緒丹飾)</h3>
<img src="images/02.jpg" alt="02 jpg" class="widget-image">
</div>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">顧筱柒 (關芯飾)</h3>
<img src="images/03.jpg" alt="03 jpg" class="widget-image">
</div>
</div>
</aside>
</div>
<footer>
<p></p>
<p></p>
</footer>
</body>
</html>
CSS
/* Fonts
-----
font-family: 'Lora', serif;
font-family: 'Ubuntu', sans-serif;
*/
body {
margin: 0;
font-family: 'Ubuntu', sans-serif;
font-size: 1.125rem;
font-weight: 300;
}
img {
max-width: 100%;
display: block;
}
/* =================
Typography
================= */
h1,
h2,
h3 {
font-family: 'Lora', serif;
font-weight: 400;
color: #143774;
margin-top: 0;
}
h1 {
font-size: 2rem;
margin: 0;
}
a {
color: #1792d2;
}
a:hover,
a:focus {
color: #143774;
}
strong {
font-weight: 700;
}
/* h1 subtitle */
.subtitle {
font-weight: 700;
color: #1792d2;
font-size: 0.75rem;
margin: 0;
}
.article-title {
font-size: 1.5rem;
}
.article-read-more,
.article-info {
font-size: 0.875rem;
}
.article-read-more {
color: #1792d2;
text-decoration: none;
font-weight: 700;
}
.article-read-more:hover,
.article-read-more:focus {
color: #143774;
text-decoration: underline;
}
.article-info {
margin: 2em 0;
}
/* =================
Layout
================= */
.container {
width: 90%;
max-width: 900px;
margin: 0 auto;
}
.container-flex {
display: flex;
flex-direction: column;
justify-content: space-between;
}
header {
background: #f8f8f8;
padding: 2em 0;
text-align: center;
}
@media (min-width: 675px) {
.container-flex {
flex-direction: row;
}
main {
width: 75%;
}
aside {
width: 20%;
}
}
/* navigation */
nav ul {
list-style: none;
padding: 0;
display: flex;
justify-content: center;
}
nav li {
margin-left: 2em;
}
nav a {
text-decoration: none;
color: #707070;
font-weight: 700;
padding: 0.25em 0;
}
nav a:hover,
nav a:focus {
color: #1792d2;
}
.current-page {
border-bottom: 1px solid #707070;
}
.current-page:hover {
color: #707070;
}
@media (max-width: 675px) {
nav ul {
flex-direction: column;
}
nav li {
margin: 0.5em 0;
}
}
/* articles */
.article-featured {
border-bottom: 1px solid #707070;
padding-bottom: 2em;
margin-bottom: 2em;
}
.article-recent {
display: flex;
flex-direction: column;
margin-bottom: 2em;
}
.article-recent-main {
order: 2;
}
.article-recent-secondary {
order: 1;
}
@media (min-width: 550px) {
.article-recent-main {
margin-top: -2.5em;
}
.article-info {
text-align: right;
}
}
37. Changing the visual order with flex box
程式碼範例
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>intro to rwd</title>
<link href="https://fonts.googleapis.com/css2?family=Lora&family=Ubuntu:wght@300;400;700&display=swap" rel="stylesheet">
<link rel="stylesheet" href="css/all.css">
</head>
<body>
<header>
<div class="container container-flex">
<div class="site-title">
<h1>程序員那麼可愛</h1>
<p class="subtitle">Cute Programmer</p>
</div>
<nav>
<ul>
<li><a href="#" class="current-page">首頁</a></li>
<li><a href="#">關於愛情</a></li>
<li><a href="#">劇情介紹</a></li>
</ul>
</nav>
</div> <!-- / .container -->
</header>
<div class="container container-flex">
<main role="main">
<article class="article-featured">
<h2 class="article-title">劇情介紹</h2>
<img src="images/banner.jpg" alt="banner jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
<p class="article-body">陸漓(<a href="#">祝緒丹</a>飾)是女程式設計師。為了追求理想而投身編程領域,她憑藉自身經歷及過人的智慧搞定學長姜逸城(<a href="#">邢昭林</a>飾),擺平他無數次的相親後,成功進入異承公司,勇闖IT世界。倆人因程序代碼結緣,後在機緣巧合下成為同居的室友。性格迥異的他們在同居相處中鬥智鬥勇情況下,<strong>是否會意外觸發心動的代碼?</strong></p>
<a href="#" class="article-read-more">繼續閱讀</a>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">姜逸城 (邢昭林飾)</h2>
<p class="article-body">28歲,陸漓的學長。性格傲嬌又自戀的霸道總裁。聰明的他是個名副其實的毒舌。喜歡在完全不講道理的情況下講道理。雖然懶散,但他是信奉效率和結果至上主義者,對員工要求極高。後在機緣巧合下成為陸漓的同居室友。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/01.jpg" alt="01 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">陸漓 (祝緒丹飾)</h2>
<p class="article-body">22歲,可愛學霸。暗戀姜逸城,是他同科系的學妹,曾幫他擺平無數次的相親。為了接近他而決定當個女程式設計師,經歷過無數關卡,甚至還女扮男裝,努力進入不接受收女程式設計師的異承公司。後在機緣巧合下成為姜逸城的同居室友。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/02.jpg" alt="02 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">顧筱柒 (關芯飾)</h2>
<p class="article-body">別名小七。陸漓的頭號閨蜜,二次元服裝網店的老闆。熱情活潑、精力充沛。遇到帥哥時,立馬化身為撩漢魔女,但每次戀愛總莫名無疾而終。性格馬虎,常常鬧出笑話來。與顧默是青梅竹馬,乾親至交。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/03.jpg" alt="03 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
</main>
<aside class="sidebar">
<div class="sidebar-widget">
<h2 class="widget-title">導語</h2>
<img src="images/aboutme.jpg" alt="aboutme jpg" class="widget-image">
<p class="widget-body">
為了自己的暗戀事業和人生目標,陸漓拼盡一切抓住機會,終於和姜逸城達成一年的“契約夫婦”。兩人在同居中展開甜蜜互動,姜逸城對陸漓漸漸動心而不自知……
</p>
</div>
<div class="sidebar-widget">
<h2 class="widget-title">最近文章</h2>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">姜逸城 (邢昭林飾)</h3>
<img src="images/01.jpg" alt="01 jpg" class="widget-image">
</div>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">陸漓 (祝緒丹飾)</h3>
<img src="images/02.jpg" alt="02 jpg" class="widget-image">
</div>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">顧筱柒 (關芯飾)</h3>
<img src="images/03.jpg" alt="03 jpg" class="widget-image">
</div>
</div>
</aside>
</div>
<footer>
<p></p>
<p></p>
</footer>
</body>
</html>
CSS
/* Fonts
-----
font-family: 'Lora', serif;
font-family: 'Ubuntu', sans-serif;
*/
body {
margin: 0;
font-family: 'Ubuntu', sans-serif;
font-size: 1.125rem;
font-weight: 300;
}
img {
max-width: 100%;
display: block;
}
/* =================
Typography
================= */
h1,
h2,
h3 {
font-family: 'Lora', serif;
font-weight: 400;
color: #143774;
margin-top: 0;
}
h1 {
font-size: 2rem;
margin: 0;
}
a {
color: #1792d2;
}
a:hover,
a:focus {
color: #143774;
}
strong {
font-weight: 700;
}
/* h1 subtitle */
.subtitle {
font-weight: 700;
color: #1792d2;
font-size: 0.75rem;
margin: 0;
}
.article-title {
font-size: 1.5rem;
}
.article-read-more,
.article-info {
font-size: 0.875rem;
}
.article-read-more {
color: #1792d2;
text-decoration: none;
font-weight: 700;
}
.article-read-more:hover,
.article-read-more:focus {
color: #143774;
text-decoration: underline;
}
.article-info {
margin: 2em 0;
}
/* =================
Layout
================= */
.container {
width: 90%;
max-width: 900px;
margin: 0 auto;
}
.container-flex {
display: flex;
flex-direction: column;
justify-content: space-between;
}
header {
background: #f8f8f8;
padding: 2em 0;
text-align: center;
}
@media (min-width: 675px) {
.container-flex {
flex-direction: row;
}
main {
width: 75%;
}
aside {
width: 20%;
}
}
/* navigation */
nav ul {
list-style: none;
padding: 0;
display: flex;
justify-content: center;
}
nav li {
margin-left: 2em;
}
nav a {
text-decoration: none;
color: #707070;
font-weight: 700;
padding: 0.25em 0;
}
nav a:hover,
nav a:focus {
color: #1792d2;
}
.current-page {
border-bottom: 1px solid #707070;
}
.current-page:hover {
color: #707070;
}
@media (max-width: 675px) {
nav ul {
flex-direction: column;
}
nav li {
margin: 0.5em 0;
}
}
/* articles */
.article-featured {
border-bottom: 1px solid #707070;
padding-bottom: 2em;
margin-bottom: 2em;
}
.article-recent {
display: flex;
flex-direction: column;
margin-bottom: 2em;
}
.article-recent-main {
order: 2;
}
.article-recent-secondary {
order: 1;
}
@media (min-width: 675px) {
.article-recent {
flex-direction: row;
justify-content: space-between;
}
.article-recent-main {
width: 68%;
}
.article-recent-secondary {
width: 30%;
}
.article-image {
width: 100%;
min-height: 250px;
object-fit: cover;
/* object-position: left; */
}
}
自我補充:object-fit – MDN Web Docs
38. Styling recent articles for large screens
程式碼範例
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>intro to rwd</title>
<link href="https://fonts.googleapis.com/css2?family=Lora&family=Ubuntu:wght@300;400;700&display=swap" rel="stylesheet">
<link rel="stylesheet" href="css/all.css">
</head>
<body>
<header>
<div class="container container-flex">
<div class="site-title">
<h1>程序員那麼可愛</h1>
<p class="subtitle">Cute Programmer</p>
</div>
<nav>
<ul>
<li><a href="#" class="current-page">首頁</a></li>
<li><a href="#">關於愛情</a></li>
<li><a href="#">劇情介紹</a></li>
</ul>
</nav>
</div> <!-- / .container -->
</header>
<div class="container container-flex">
<main role="main">
<article class="article-featured">
<h2 class="article-title">劇情介紹</h2>
<img src="images/banner.jpg" alt="banner jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
<p class="article-body">陸漓(<a href="#">祝緒丹</a>飾)是女程式設計師。為了追求理想而投身編程領域,她憑藉自身經歷及過人的智慧搞定學長姜逸城(<a href="#">邢昭林</a>飾),擺平他無數次的相親後,成功進入異承公司,勇闖IT世界。倆人因程序代碼結緣,後在機緣巧合下成為同居的室友。性格迥異的他們在同居相處中鬥智鬥勇情況下,<strong>是否會意外觸發心動的代碼?</strong></p>
<a href="#" class="article-read-more">繼續閱讀</a>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">姜逸城 (邢昭林飾)</h2>
<p class="article-body">28歲,陸漓的學長。性格傲嬌又自戀的霸道總裁。聰明的他是個名副其實的毒舌。喜歡在完全不講道理的情況下講道理。雖然懶散,但他是信奉效率和結果至上主義者,對員工要求極高。後在機緣巧合下成為陸漓的同居室友。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/01.jpg" alt="01 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">陸漓 (祝緒丹飾)</h2>
<p class="article-body">22歲,可愛學霸。暗戀姜逸城,是他同科系的學妹,曾幫他擺平無數次的相親。為了接近他而決定當個女程式設計師,經歷過無數關卡,甚至還女扮男裝,努力進入不接受收女程式設計師的異承公司。後在機緣巧合下成為姜逸城的同居室友。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/02.jpg" alt="02 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">顧筱柒 (關芯飾)</h2>
<p class="article-body">別名小七。陸漓的頭號閨蜜,二次元服裝網店的老闆。熱情活潑、精力充沛。遇到帥哥時,立馬化身為撩漢魔女,但每次戀愛總莫名無疾而終。性格馬虎,常常鬧出笑話來。與顧默是青梅竹馬,乾親至交。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/03.jpg" alt="03 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
</main>
<aside class="sidebar">
<div class="sidebar-widget">
<h2 class="widget-title">導語</h2>
<img src="images/aboutme.jpg" alt="aboutme jpg" class="widget-image">
<p class="widget-body">
為了自己的暗戀事業和人生目標,陸漓拼盡一切抓住機會,終於和姜逸城達成一年的“契約夫婦”。兩人在同居中展開甜蜜互動,姜逸城對陸漓漸漸動心而不自知……
</p>
</div>
<div class="sidebar-widget">
<h2 class="widget-title">最近文章</h2>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">姜逸城 (邢昭林飾)</h3>
<img src="images/01.jpg" alt="01 jpg" class="widget-image">
</div>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">陸漓 (祝緒丹飾)</h3>
<img src="images/02.jpg" alt="02 jpg" class="widget-image">
</div>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">顧筱柒 (關芯飾)</h3>
<img src="images/03.jpg" alt="03 jpg" class="widget-image">
</div>
</div>
</aside>
</div>
<footer>
<p></p>
<p></p>
</footer>
</body>
</html>
CSS
/* Fonts
-----
font-family: 'Lora', serif;
font-family: 'Ubuntu', sans-serif;
*/
body {
margin: 0;
font-family: 'Ubuntu', sans-serif;
font-size: 1.125rem;
font-weight: 300;
}
img {
max-width: 100%;
display: block;
}
/* =================
Typography
================= */
h1,
h2,
h3 {
font-family: 'Lora', serif;
font-weight: 400;
color: #143774;
margin-top: 0;
}
h1 {
font-size: 2rem;
margin: 0;
}
a {
color: #1792d2;
}
a:hover,
a:focus {
color: #143774;
}
strong {
font-weight: 700;
}
/* h1 subtitle */
.subtitle {
font-weight: 700;
color: #1792d2;
font-size: 0.75rem;
margin: 0;
}
.article-title {
font-size: 1.5rem;
}
.article-read-more,
.article-info {
font-size: 0.875rem;
}
.article-read-more {
color: #1792d2;
text-decoration: none;
font-weight: 700;
}
.article-read-more:hover,
.article-read-more:focus {
color: #143774;
text-decoration: underline;
}
.article-info {
margin: 2em 0;
}
/* =================
Layout
================= */
.container {
width: 90%;
max-width: 900px;
margin: 0 auto;
}
.container-flex {
display: flex;
flex-direction: column;
justify-content: space-between;
}
header {
background: #f8f8f8;
padding: 2em 0;
text-align: center;
margin-bottom: 3em;
}
@media (min-width: 675px) {
.container-flex {
flex-direction: row;
}
main {
width: 75%;
}
aside {
width: 20%;
}
}
/* navigation */
nav ul {
list-style: none;
padding: 0;
display: flex;
justify-content: center;
}
nav li {
margin-left: 2em;
}
nav a {
text-decoration: none;
color: #707070;
font-weight: 700;
padding: 0.25em 0;
}
nav a:hover,
nav a:focus {
color: #1792d2;
}
.current-page {
border-bottom: 1px solid #707070;
}
.current-page:hover {
color: #707070;
}
@media (max-width: 675px) {
nav ul {
flex-direction: column;
}
nav li {
margin: 0.5em 0;
}
}
/* articles */
.article-featured {
border-bottom: 1px solid #707070;
padding-bottom: 2em;
margin-bottom: 2em;
}
.article-recent {
display: flex;
flex-direction: column;
margin-bottom: 2em;
}
.article-recent-main {
order: 2;
}
.article-recent-secondary {
order: 1;
}
@media (min-width: 675px) {
.article-recent {
flex-direction: row;
justify-content: space-between;
}
.article-recent-main {
width: 68%;
}
.article-recent-secondary {
width: 30%;
}
.article-featured {
display: flex;
flex-direction: column;
}
.article-image {
order: -2;
}
.article-info {
order: -1;
}
}
39. Setting up the widgets and talking breakpoints
程式碼範例
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>intro to rwd</title>
<link href="https://fonts.googleapis.com/css2?family=Lora&family=Ubuntu:wght@300;400;700&display=swap" rel="stylesheet">
<link rel="stylesheet" href="css/all.css">
</head>
<body>
<header>
<div class="container container-flex">
<div class="site-title">
<h1>程序員那麼可愛</h1>
<p class="subtitle">Cute Programmer</p>
</div>
<nav>
<ul>
<li><a href="#" class="current-page">首頁</a></li>
<li><a href="#">關於愛情</a></li>
<li><a href="#">劇情介紹</a></li>
</ul>
</nav>
</div> <!-- / .container -->
</header>
<div class="container container-flex">
<main role="main">
<article class="article-featured">
<h2 class="article-title">劇情介紹</h2>
<img src="images/banner.jpg" alt="banner jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
<p class="article-body">陸漓(<a href="#">祝緒丹</a>飾)是女程式設計師。為了追求理想而投身編程領域,她憑藉自身經歷及過人的智慧搞定學長姜逸城(<a href="#">邢昭林</a>飾),擺平他無數次的相親後,成功進入異承公司,勇闖IT世界。倆人因程序代碼結緣,後在機緣巧合下成為同居的室友。性格迥異的他們在同居相處中鬥智鬥勇情況下,<strong>是否會意外觸發心動的代碼?</strong></p>
<a href="#" class="article-read-more">繼續閱讀</a>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">姜逸城 (邢昭林飾)</h2>
<p class="article-body">28歲,陸漓的學長。性格傲嬌又自戀的霸道總裁。聰明的他是個名副其實的毒舌。喜歡在完全不講道理的情況下講道理。雖然懶散,但他是信奉效率和結果至上主義者,對員工要求極高。後在機緣巧合下成為陸漓的同居室友。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/01.jpg" alt="01 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">陸漓 (祝緒丹飾)</h2>
<p class="article-body">22歲,可愛學霸。暗戀姜逸城,是他同科系的學妹,曾幫他擺平無數次的相親。為了接近他而決定當個女程式設計師,經歷過無數關卡,甚至還女扮男裝,努力進入不接受收女程式設計師的異承公司。後在機緣巧合下成為姜逸城的同居室友。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/02.jpg" alt="02 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">顧筱柒 (關芯飾)</h2>
<p class="article-body">別名小七。陸漓的頭號閨蜜,二次元服裝網店的老闆。熱情活潑、精力充沛。遇到帥哥時,立馬化身為撩漢魔女,但每次戀愛總莫名無疾而終。性格馬虎,常常鬧出笑話來。與顧默是青梅竹馬,乾親至交。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/03.jpg" alt="03 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
</main>
<aside class="sidebar">
<div class="sidebar-widget">
<h2 class="widget-title">導語</h2>
<img src="images/aboutme.jpg" alt="aboutme jpg" class="widget-image">
<p class="widget-body">
為了自己的暗戀事業和人生目標,陸漓拼盡一切抓住機會,終於和姜逸城達成一年的“契約夫婦”。兩人在同居中展開甜蜜互動,姜逸城對陸漓漸漸動心而不自知……
</p>
</div>
<div class="sidebar-widget">
<h2 class="widget-title">最近文章</h2>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">姜逸城 (邢昭林飾)</h3>
<img src="images/01.jpg" alt="01 jpg" class="widget-image">
</div>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">陸漓 (祝緒丹飾)</h3>
<img src="images/02.jpg" alt="02 jpg" class="widget-image">
</div>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">顧筱柒 (關芯飾)</h3>
<img src="images/03.jpg" alt="03 jpg" class="widget-image">
</div>
</div>
</aside>
</div>
<footer>
<p></p>
<p></p>
</footer>
</body>
</html>
CSS
/* Fonts
-----
font-family: 'Lora', serif;
font-family: 'Ubuntu', sans-serif;
*/
body {
margin: 0;
font-family: 'Ubuntu', sans-serif;
font-size: 1.125rem;
font-weight: 300;
}
img {
max-width: 100%;
display: block;
}
/* =================
Typography
================= */
h1,
h2,
h3 {
font-family: 'Lora', serif;
font-weight: 400;
color: #143774;
margin-top: 0;
}
h1 {
font-size: 2rem;
margin: 0;
}
a {
color: #1792d2;
}
a:hover,
a:focus {
color: #143774;
}
strong {
font-weight: 700;
}
/* h1 subtitle */
.subtitle {
font-weight: 700;
color: #1792d2;
font-size: 0.75rem;
margin: 0;
}
.article-title {
font-size: 1.5rem;
}
.article-read-more,
.article-info {
font-size: 0.875rem;
}
.article-read-more {
color: #1792d2;
text-decoration: none;
font-weight: 700;
}
.article-read-more:hover,
.article-read-more:focus {
color: #143774;
text-decoration: underline;
}
.article-info {
margin: 2em 0;
}
/* =================
Layout
================= */
.container {
width: 90%;
max-width: 900px;
margin: 0 auto;
}
.container-flex {
display: flex;
flex-direction: column;
justify-content: space-between;
}
header {
background: #f8f8f8;
padding: 2em 0;
text-align: center;
margin-bottom: 3em;
}
@media (min-width: 675px) {
.container-flex {
flex-direction: row;
}
main {
width: 70%;
}
aside {
width: 25%;
min-width: 200px;
margin-left: 1em;
}
}
/* navigation */
nav ul {
list-style: none;
padding: 0;
display: flex;
justify-content: center;
}
nav li {
margin-left: 2em;
}
nav a {
text-decoration: none;
color: #707070;
font-weight: 700;
padding: 0.25em 0;
}
nav a:hover,
nav a:focus {
color: #1792d2;
}
.current-page {
border-bottom: 1px solid #707070;
}
.current-page:hover {
color: #707070;
}
@media (max-width: 675px) {
nav ul {
flex-direction: column;
}
nav li {
margin: 0.5em 0;
}
}
/* articles */
.article-featured {
border-bottom: 1px solid #707070;
padding-bottom: 2em;
margin-bottom: 2em;
}
.article-recent {
display: flex;
flex-direction: column;
margin-bottom: 2em;
}
.article-recent-main {
order: 2;
}
.article-recent-secondary {
order: 1;
}
@media (min-width: 675px) {
.article-recent {
flex-direction: row;
justify-content: space-between;
}
.article-recent-main {
width: 68%;
}
.article-recent-secondary {
width: 30%;
}
.article-featured {
display: flex;
flex-direction: column;
}
.article-image {
order: -2;
min-height: 250px;
object-fit: cover;
/* object-position: left; */
}
.article-info {
order: -1;
}
}
/* widgets */
.sidebar-widget {
border: 20px solid #ebebeb;
margin-bottom: 2em;
padding: 1em;
}
40. Using a new pseudo-class to wrap-up the homepage
程式碼範例
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>intro to rwd</title>
<link href="https://fonts.googleapis.com/css2?family=Lora&family=Ubuntu:wght@300;400;700&display=swap" rel="stylesheet">
<link rel="stylesheet" href="css/all.css">
</head>
<body>
<header>
<div class="container container-flex">
<div class="site-title">
<h1>程序員那麼可愛</h1>
<p class="subtitle">Cute Programmer</p>
</div>
<nav>
<ul>
<li><a href="#" class="current-page">首頁</a></li>
<li><a href="#">關於愛情</a></li>
<li><a href="#">劇情介紹</a></li>
</ul>
</nav>
</div> <!-- / .container -->
</header>
<div class="container container-flex">
<main role="main">
<article class="article-featured">
<h2 class="article-title">劇情介紹</h2>
<img src="images/banner.jpg" alt="banner jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
<p class="article-body">陸漓(<a href="#">祝緒丹</a>飾)是女程式設計師。為了追求理想而投身編程領域,她憑藉自身經歷及過人的智慧搞定學長姜逸城(<a href="#">邢昭林</a>飾),擺平他無數次的相親後,成功進入異承公司,勇闖IT世界。倆人因程序代碼結緣,後在機緣巧合下成為同居的室友。性格迥異的他們在同居相處中鬥智鬥勇情況下,<strong>是否會意外觸發心動的代碼?</strong></p>
<a href="#" class="article-read-more">繼續閱讀</a>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">姜逸城 (邢昭林飾)</h2>
<p class="article-body">28歲,陸漓的學長。性格傲嬌又自戀的霸道總裁。聰明的他是個名副其實的毒舌。喜歡在完全不講道理的情況下講道理。雖然懶散,但他是信奉效率和結果至上主義者,對員工要求極高。後在機緣巧合下成為陸漓的同居室友。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/01.jpg" alt="01 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">陸漓 (祝緒丹飾)</h2>
<p class="article-body">22歲,可愛學霸。暗戀姜逸城,是他同科系的學妹,曾幫他擺平無數次的相親。為了接近他而決定當個女程式設計師,經歷過無數關卡,甚至還女扮男裝,努力進入不接受收女程式設計師的異承公司。後在機緣巧合下成為姜逸城的同居室友。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/02.jpg" alt="02 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">顧筱柒 (關芯飾)</h2>
<p class="article-body">別名小七。陸漓的頭號閨蜜,二次元服裝網店的老闆。熱情活潑、精力充沛。遇到帥哥時,立馬化身為撩漢魔女,但每次戀愛總莫名無疾而終。性格馬虎,常常鬧出笑話來。與顧默是青梅竹馬,乾親至交。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/03.jpg" alt="03 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
</main>
<aside class="sidebar">
<div class="sidebar-widget">
<h2 class="widget-title">導語</h2>
<img src="images/aboutme.jpg" alt="aboutme jpg" class="widget-image">
<p class="widget-body">
為了自己的暗戀事業和人生目標,陸漓拼盡一切抓住機會,終於和姜逸城達成一年的“契約夫婦”。兩人在同居中展開甜蜜互動,姜逸城對陸漓漸漸動心而不自知……
</p>
</div>
<div class="sidebar-widget">
<h2 class="widget-title">最近文章</h2>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">姜逸城 (邢昭林飾)</h3>
<img src="images/01.jpg" alt="01 jpg" class="widget-image">
</div>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">陸漓 (祝緒丹飾)</h3>
<img src="images/02.jpg" alt="02 jpg" class="widget-image">
</div>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">顧筱柒 (關芯飾)</h3>
<img src="images/03.jpg" alt="03 jpg" class="widget-image">
</div>
</div>
</aside>
</div>
<footer>
<p><strong>非正式官網,網站僅供練習使用。</strong></p>
<p>程序員那麼可愛</p>
</footer>
</body>
</html>
CSS
/* Fonts
-----
font-family: 'Lora', serif;
font-family: 'Ubuntu', sans-serif;
*/
body {
margin: 0;
font-family: 'Ubuntu', sans-serif;
font-size: 1.125rem;
font-weight: 300;
}
img {
max-width: 100%;
display: block;
}
/* =================
Typography
================= */
h1,
h2,
h3 {
font-family: 'Lora', serif;
font-weight: 400;
color: #143774;
margin-top: 0;
}
h1 {
font-size: 2rem;
margin: 0;
}
a {
color: #1792d2;
}
a:hover,
a:focus {
color: #143774;
}
strong {
font-weight: 700;
}
/* h1 subtitle */
.subtitle {
font-weight: 700;
color: #1792d2;
font-size: 0.75rem;
margin: 0;
}
.article-title {
font-size: 1.5rem;
}
.article-read-more,
.article-info {
font-size: 0.875rem;
}
.article-read-more {
color: #1792d2;
text-decoration: none;
font-weight: 700;
}
.article-read-more:hover,
.article-read-more:focus {
color: #143774;
text-decoration: underline;
}
.article-info {
margin: 2em 0;
}
.widget-title,
.widget-recent-post-title {
font-size: 1rem;
}
.widget-title {
font-family: 'Ubuntu', sans-serif;
font-weight: 700;
}
/* =================
Layout
================= */
.container {
width: 90%;
max-width: 900px;
margin: 0 auto;
}
.container-flex {
display: flex;
flex-direction: column;
justify-content: space-between;
}
header {
background: #f8f8f8;
padding: 2em 0;
text-align: center;
margin-bottom: 3em;
}
footer {
background: #143774;
color: #fff;
text-align: center;
padding: 3em 0;
}
@media (min-width: 675px) {
.container-flex {
flex-direction: row;
}
main {
width: 70%;
}
aside {
width: 25%;
min-width: 200px;
margin-left: 1em;
}
}
/* navigation */
nav ul {
list-style: none;
padding: 0;
display: flex;
justify-content: center;
}
nav li {
margin-left: 2em;
}
nav a {
text-decoration: none;
color: #707070;
font-weight: 700;
padding: 0.25em 0;
}
nav a:hover,
nav a:focus {
color: #1792d2;
}
.current-page {
border-bottom: 1px solid #707070;
}
.current-page:hover {
color: #707070;
}
@media (max-width: 675px) {
nav ul {
flex-direction: column;
}
nav li {
margin: 0.5em 0;
}
}
/* articles */
.article-featured {
border-bottom: 1px solid #707070;
padding-bottom: 2em;
margin-bottom: 2em;
}
.article-recent {
display: flex;
flex-direction: column;
margin-bottom: 2em;
}
.article-recent-main {
order: 2;
}
.article-recent-secondary {
order: 1;
}
@media (min-width: 675px) {
.article-recent {
flex-direction: row;
justify-content: space-between;
}
.article-recent-main {
width: 68%;
}
.article-recent-secondary {
width: 30%;
}
.article-featured {
display: flex;
flex-direction: column;
}
.article-image {
order: -2;
min-height: 250px;
object-fit: cover;
/* object-position: left; */
}
.article-info {
order: -1;
}
}
/* widgets */
.sidebar-widget {
border: 20px solid #ebebeb;
margin-bottom: 2em;
padding: 1em;
}
.widget-recent-post {
display: flex;
flex-direction: column;
border-bottom: 1px solid #707070;
margin-bottom: 1em;
}
.widget-recent-post:last-child {
border: 0;
margin: 0;
}
.widget-image {
order: -1;
}
41. Creating the recent posts page
程式碼範例
HTML – index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>intro to rwd</title>
<link href="https://fonts.googleapis.com/css2?family=Lora&family=Ubuntu:wght@300;400;700&display=swap" rel="stylesheet">
<link rel="stylesheet" href="css/all.css">
</head>
<body>
<header>
<div class="container container-flex">
<div class="site-title">
<h1>程序員那麼可愛</h1>
<p class="subtitle">Cute Programmer</p>
</div>
<nav>
<ul>
<li><a href="index.html">首頁</a></li>
<li><a href="#">關於愛情</a></li>
<li><a href="recent-posts.html">劇情介紹</a></li>
</ul>
</nav>
</div> <!-- / .container -->
</header>
<div class="container container-flex">
<main role="main">
<article class="article-featured">
<h2 class="article-title">劇情介紹</h2>
<img src="images/banner.jpg" alt="banner jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
<p class="article-body">陸漓(<a href="#">祝緒丹</a>飾)是女程式設計師。為了追求理想而投身編程領域,她憑藉自身經歷及過人的智慧搞定學長姜逸城(<a href="#">邢昭林</a>飾),擺平他無數次的相親後,成功進入異承公司,勇闖IT世界。倆人因程序代碼結緣,後在機緣巧合下成為同居的室友。性格迥異的他們在同居相處中鬥智鬥勇情況下,<strong>是否會意外觸發心動的代碼?</strong></p>
<a href="#" class="article-read-more">繼續閱讀</a>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">姜逸城 (邢昭林飾)</h2>
<p class="article-body">28歲,陸漓的學長。性格傲嬌又自戀的霸道總裁。聰明的他是個名副其實的毒舌。喜歡在完全不講道理的情況下講道理。雖然懶散,但他是信奉效率和結果至上主義者,對員工要求極高。後在機緣巧合下成為陸漓的同居室友。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/01.jpg" alt="01 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">陸漓 (祝緒丹飾)</h2>
<p class="article-body">22歲,可愛學霸。暗戀姜逸城,是他同科系的學妹,曾幫他擺平無數次的相親。為了接近他而決定當個女程式設計師,經歷過無數關卡,甚至還女扮男裝,努力進入不接受收女程式設計師的異承公司。後在機緣巧合下成為姜逸城的同居室友。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/02.jpg" alt="02 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">顧筱柒 (關芯飾)</h2>
<p class="article-body">別名小七。陸漓的頭號閨蜜,二次元服裝網店的老闆。熱情活潑、精力充沛。遇到帥哥時,立馬化身為撩漢魔女,但每次戀愛總莫名無疾而終。性格馬虎,常常鬧出笑話來。與顧默是青梅竹馬,乾親至交。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/03.jpg" alt="03 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
</main>
<aside class="sidebar">
<div class="sidebar-widget">
<h2 class="widget-title">導語</h2>
<img src="images/aboutme.jpg" alt="aboutme jpg" class="widget-image">
<p class="widget-body">
為了自己的暗戀事業和人生目標,陸漓拼盡一切抓住機會,終於和姜逸城達成一年的“契約夫婦”。兩人在同居中展開甜蜜互動,姜逸城對陸漓漸漸動心而不自知……
</p>
</div>
<div class="sidebar-widget">
<h2 class="widget-title">最近文章</h2>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">姜逸城 (邢昭林飾)</h3>
<img src="images/01.jpg" alt="01 jpg" class="widget-image">
</div>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">陸漓 (祝緒丹飾)</h3>
<img src="images/02.jpg" alt="02 jpg" class="widget-image">
</div>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">顧筱柒 (關芯飾)</h3>
<img src="images/03.jpg" alt="03 jpg" class="widget-image">
</div>
</div>
</aside>
</div>
<footer>
<p><strong>非正式官網,網站僅供練習使用。</strong></p>
<p>程序員那麼可愛</p>
</footer>
</body>
</html>
HTML – recent-posts.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>intro to rwd</title>
<link href="https://fonts.googleapis.com/css2?family=Lora&family=Ubuntu:wght@300;400;700&display=swap" rel="stylesheet">
<link rel="stylesheet" href="css/all.css">
</head>
<body>
<header>
<div class="container container-flex">
<div class="site-title">
<h1>程序員那麼可愛</h1>
<p class="subtitle">Cute Programmer</p>
</div>
<nav>
<ul>
<li><a href="index.html">首頁</a></li>
<li><a href="#">關於愛情</a></li>
<li><a href="recent-posts.html">劇情介紹</a></li>
</ul>
</nav>
</div> <!-- / .container -->
</header>
<div class="container container-flex">
<main role="main">
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">劇情介紹</h2>
<p class="article-body">陸漓(<a href="#">祝緒丹</a>飾)是女程式設計師。為了追求理想而投身編程領域,她憑藉自身經歷及過人的智慧搞定學長姜逸城(<a href="#">邢昭林</a>飾),擺平他無數次的相親後,成功進入異承公司,勇闖IT世界。倆人因程序代碼結緣,後在機緣巧合下成為同居的室友。性格迥異的他們在同居相處中鬥智鬥勇情況下,<strong>是否會意外觸發心動的代碼?</strong></p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/banner.jpg" alt="banner jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">姜逸城 (邢昭林飾)</h2>
<p class="article-body">28歲,陸漓的學長。性格傲嬌又自戀的霸道總裁。聰明的他是個名副其實的毒舌。喜歡在完全不講道理的情況下講道理。雖然懶散,但他是信奉效率和結果至上主義者,對員工要求極高。後在機緣巧合下成為陸漓的同居室友。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/01.jpg" alt="01 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">陸漓 (祝緒丹飾)</h2>
<p class="article-body">22歲,可愛學霸。暗戀姜逸城,是他同科系的學妹,曾幫他擺平無數次的相親。為了接近他而決定當個女程式設計師,經歷過無數關卡,甚至還女扮男裝,努力進入不接受收女程式設計師的異承公司。後在機緣巧合下成為姜逸城的同居室友。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/02.jpg" alt="02 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">顧筱柒 (關芯飾)</h2>
<p class="article-body">別名小七。陸漓的頭號閨蜜,二次元服裝網店的老闆。熱情活潑、精力充沛。遇到帥哥時,立馬化身為撩漢魔女,但每次戀愛總莫名無疾而終。性格馬虎,常常鬧出笑話來。與顧默是青梅竹馬,乾親至交。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/03.jpg" alt="03 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
</main>
<aside class="sidebar">
<div class="sidebar-widget">
<h2 class="widget-title">導語</h2>
<img src="images/aboutme.jpg" alt="aboutme jpg" class="widget-image">
<p class="widget-body">
為了自己的暗戀事業和人生目標,陸漓拼盡一切抓住機會,終於和姜逸城達成一年的“契約夫婦”。兩人在同居中展開甜蜜互動,姜逸城對陸漓漸漸動心而不自知……
</p>
</div>
<div class="sidebar-widget">
<h2 class="widget-title">最近文章</h2>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">姜逸城 (邢昭林飾)</h3>
<img src="images/01.jpg" alt="01 jpg" class="widget-image">
</div>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">陸漓 (祝緒丹飾)</h3>
<img src="images/02.jpg" alt="02 jpg" class="widget-image">
</div>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">顧筱柒 (關芯飾)</h3>
<img src="images/03.jpg" alt="03 jpg" class="widget-image">
</div>
</div>
</aside>
</div>
<footer>
<p><strong>非正式官網,網站僅供練習使用。</strong></p>
<p>程序員那麼可愛</p>
</footer>
</body>
</html>
CSS
/* Fonts
-----
font-family: 'Lora', serif;
font-family: 'Ubuntu', sans-serif;
*/
body {
margin: 0;
font-family: 'Ubuntu', sans-serif;
font-size: 1.125rem;
font-weight: 300;
}
img {
max-width: 100%;
display: block;
}
/* =================
Typography
================= */
h1,
h2,
h3 {
font-family: 'Lora', serif;
font-weight: 400;
color: #143774;
margin-top: 0;
}
h1 {
font-size: 2rem;
margin: 0;
}
a {
color: #1792d2;
}
a:hover,
a:focus {
color: #143774;
}
strong {
font-weight: 700;
}
/* h1 subtitle */
.subtitle {
font-weight: 700;
color: #1792d2;
font-size: 0.75rem;
margin: 0;
}
.article-title {
font-size: 1.5rem;
}
.article-read-more,
.article-info {
font-size: 0.875rem;
}
.article-read-more {
color: #1792d2;
text-decoration: none;
font-weight: 700;
}
.article-read-more:hover,
.article-read-more:focus {
color: #143774;
text-decoration: underline;
}
.article-info {
margin: 2em 0;
}
.widget-title,
.widget-recent-post-title {
font-size: 1rem;
}
.widget-title {
font-family: 'Ubuntu', sans-serif;
font-weight: 700;
}
/* =================
Layout
================= */
.container {
width: 90%;
max-width: 900px;
margin: 0 auto;
}
.container-flex {
display: flex;
flex-direction: column;
justify-content: space-between;
}
header {
background: #f8f8f8;
padding: 2em 0;
text-align: center;
margin-bottom: 3em;
}
footer {
background: #143774;
color: #fff;
text-align: center;
padding: 3em 0;
}
@media (min-width: 675px) {
.container-flex {
flex-direction: row;
}
main {
width: 70%;
}
aside {
width: 25%;
min-width: 200px;
margin-left: 1em;
}
}
/* navigation */
nav ul {
list-style: none;
padding: 0;
display: flex;
justify-content: center;
}
nav li {
margin-left: 2em;
}
nav a {
text-decoration: none;
color: #707070;
font-weight: 700;
padding: 0.25em 0;
}
nav a:hover,
nav a:focus {
color: #1792d2;
}
@media (max-width: 675px) {
nav ul {
flex-direction: column;
}
nav li {
margin: 0.5em 0;
}
}
/* articles */
.article-featured {
border-bottom: 1px solid #707070;
padding-bottom: 2em;
margin-bottom: 2em;
}
.article-recent {
display: flex;
flex-direction: column;
margin-bottom: 2em;
}
.article-recent-main {
order: 2;
}
.article-recent-secondary {
order: 1;
}
@media (min-width: 675px) {
.article-recent {
flex-direction: row;
justify-content: space-between;
}
.article-recent-main {
width: 68%;
}
.article-recent-secondary {
width: 30%;
}
.article-featured {
display: flex;
flex-direction: column;
}
.article-image {
order: -2;
min-height: 250px;
object-fit: cover;
/* object-position: left; */
}
.article-info {
order: -1;
}
}
/* widgets */
.sidebar-widget {
border: 20px solid #ebebeb;
margin-bottom: 2em;
padding: 1em;
}
.widget-recent-post {
display: flex;
flex-direction: column;
border-bottom: 1px solid #707070;
margin-bottom: 1em;
}
.widget-recent-post:last-child {
border: 0;
margin: 0;
}
.widget-image {
order: -1;
}
42. Setting up the About Me page
程式碼範例
HTML – index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>intro to rwd</title>
<link rel="shortcut icon" href="images/cute-programmer.jpg" type="image/x-icon">
<link href="https://fonts.googleapis.com/css2?family=Lora&family=Ubuntu:wght@300;400;700&display=swap" rel="stylesheet">
<link rel="stylesheet" href="css/all.css">
</head>
<body>
<header>
<div class="container container-flex">
<div class="site-title">
<h1>程序員那麼可愛</h1>
<p class="subtitle">Cute Programmer</p>
</div>
<nav>
<ul>
<li><a href="index.html">首頁</a></li>
<li><a href="about-love.html">關於愛情</a></li>
<li><a href="recent-posts.html">劇情介紹</a></li>
</ul>
</nav>
</div> <!-- / .container -->
</header>
<div class="container container-flex">
<main role="main">
<article class="article-featured">
<h2 class="article-title">劇情介紹</h2>
<img src="images/banner.jpg" alt="banner jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
<p class="article-body">陸漓(<a href="#">祝緒丹</a>飾)是女程式設計師。為了追求理想而投身編程領域,她憑藉自身經歷及過人的智慧搞定學長姜逸城(<a href="#">邢昭林</a>飾),擺平他無數次的相親後,成功進入異承公司,勇闖IT世界。倆人因程序代碼結緣,後在機緣巧合下成為同居的室友。性格迥異的他們在同居相處中鬥智鬥勇情況下,<strong>是否會意外觸發心動的代碼?</strong></p>
<a href="#" class="article-read-more">繼續閱讀</a>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">姜逸城 (邢昭林飾)</h2>
<p class="article-body">28歲,陸漓的學長。性格傲嬌又自戀的霸道總裁。聰明的他是個名副其實的毒舌。喜歡在完全不講道理的情況下講道理。雖然懶散,但他是信奉效率和結果至上主義者,對員工要求極高。後在機緣巧合下成為陸漓的同居室友。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/01.jpg" alt="01 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">陸漓 (祝緒丹飾)</h2>
<p class="article-body">22歲,可愛學霸。暗戀姜逸城,是他同科系的學妹,曾幫他擺平無數次的相親。為了接近他而決定當個女程式設計師,經歷過無數關卡,甚至還女扮男裝,努力進入不接受收女程式設計師的異承公司。後在機緣巧合下成為姜逸城的同居室友。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/02.jpg" alt="02 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">顧筱柒 (關芯飾)</h2>
<p class="article-body">別名小七。陸漓的頭號閨蜜,二次元服裝網店的老闆。熱情活潑、精力充沛。遇到帥哥時,立馬化身為撩漢魔女,但每次戀愛總莫名無疾而終。性格馬虎,常常鬧出笑話來。與顧默是青梅竹馬,乾親至交。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/03.jpg" alt="03 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
</main>
<aside class="sidebar">
<div class="sidebar-widget">
<h2 class="widget-title">導語</h2>
<img src="images/about-love.jpg" alt="about-love jpg" class="widget-image">
<p class="widget-body">
為了自己的暗戀事業和人生目標,陸漓拼盡一切抓住機會,終於和姜逸城達成一年的“契約夫婦”。兩人在同居中展開甜蜜互動,姜逸城對陸漓漸漸動心而不自知……
</p>
</div>
<div class="sidebar-widget">
<h2 class="widget-title">最近文章</h2>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">姜逸城 (邢昭林飾)</h3>
<img src="images/01.jpg" alt="01 jpg" class="widget-image">
</div>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">陸漓 (祝緒丹飾)</h3>
<img src="images/02.jpg" alt="02 jpg" class="widget-image">
</div>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">顧筱柒 (關芯飾)</h3>
<img src="images/03.jpg" alt="03 jpg" class="widget-image">
</div>
</div>
</aside>
</div>
<footer>
<p><strong>非正式官網,網站僅供練習使用。</strong></p>
<p>程序員那麼可愛</p>
</footer>
</body>
</html>
HTML – about-love.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>intro to rwd</title>
<link rel="shortcut icon" href="images/cute-programmer.jpg" type="image/x-icon">
<link href="https://fonts.googleapis.com/css2?family=Lora&family=Ubuntu:wght@300;400;700&display=swap" rel="stylesheet">
<link rel="stylesheet" href="css/all.css">
</head>
<body>
<header>
<div class="container container-flex">
<div class="site-title">
<h1>程序員那麼可愛</h1>
<p class="subtitle">Cute Programmer</p>
</div>
<nav>
<ul>
<li><a href="index.html">首頁</a></li>
<li><a href="about-love.html">關於愛情</a></li>
<li><a href="recent-posts.html">劇情介紹</a></li>
</ul>
</nav>
</div> <!-- / .container -->
</header>
<div class="container container-flex">
<main role="main">
<img src="images/about-love.jpg" class="image-full" alt="about-love jpg">
<h2>關於愛情</h2>
<p><strong>為了自己的暗戀事業和人生目標</strong>,陸漓拼盡一切抓住機會,終於和姜逸城達成一年的“契約夫婦”。兩人在同居中展開甜蜜互動,姜逸城對陸漓漸漸動心而不自知……</p>
<p>陸漓(祝緒丹飾)是女程式設計師。為了追求理想而投身編程領域,她憑藉自身經歷及過人的智慧搞定學長姜逸城(邢昭林飾),擺平他無數次的相親後,成功進入異承公司,勇闖IT世界。倆人因程序代碼結緣,後在機緣巧合下成為同居的室友。性格迥異的他們在同居相處中鬥智鬥勇情況下,是否會意外觸發心動的代碼?</p>
<h3>EP01 & EP02</h3>
<p>暗戀就像是等待測試的程序,不確定運行結果,也不確定運行時間,但只要需求沒變,就要努力優化程序,<strong>直到它成功運行</strong>。</p>
<p>喜歡你這件事,不會有任何BUG,所以不需要DEBUG。</p>
<h3>EP03 & EP04</h3>
<p>和暗戀的人在一起時,記得敲一遍高精度,這樣對他的喜歡,就不會溢出,被對方發現了。</p>
<p><strong>每個人都有屬於自己的秘密</strong>,雖然可以通過技術手段進行加密,但卻不知道系統何時會被黑客入侵,將我們保守的秘密公之於眾。</p>
</main>
<aside class="sidebar">
<div class="sidebar-widget">
<h2 class="widget-title">最近文章</h2>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">姜逸城 (邢昭林飾)</h3>
<img src="images/01.jpg" alt="01 jpg" class="widget-image">
</div>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">陸漓 (祝緒丹飾)</h3>
<img src="images/02.jpg" alt="02 jpg" class="widget-image">
</div>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">顧筱柒 (關芯飾)</h3>
<img src="images/03.jpg" alt="03 jpg" class="widget-image">
</div>
</div>
</aside>
</div>
<footer>
<p><strong>非正式官網,網站僅供練習使用。</strong></p>
<p>程序員那麼可愛</p>
</footer>
</body>
</html>
HTML – recent-posts.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>intro to rwd</title>
<link rel="shortcut icon" href="images/cute-programmer.jpg" type="image/x-icon">
<link href="https://fonts.googleapis.com/css2?family=Lora&family=Ubuntu:wght@300;400;700&display=swap" rel="stylesheet">
<link rel="stylesheet" href="css/all.css">
</head>
<body>
<header>
<div class="container container-flex">
<div class="site-title">
<h1>程序員那麼可愛</h1>
<p class="subtitle">Cute Programmer</p>
</div>
<nav>
<ul>
<li><a href="index.html">首頁</a></li>
<li><a href="about-love.html">關於愛情</a></li>
<li><a href="recent-posts.html">劇情介紹</a></li>
</ul>
</nav>
</div> <!-- / .container -->
</header>
<div class="container container-flex">
<main role="main">
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">劇情介紹</h2>
<p class="article-body">陸漓(<a href="#">祝緒丹</a>飾)是女程式設計師。為了追求理想而投身編程領域,她憑藉自身經歷及過人的智慧搞定學長姜逸城(<a href="#">邢昭林</a>飾),擺平他無數次的相親後,成功進入異承公司,勇闖IT世界。倆人因程序代碼結緣,後在機緣巧合下成為同居的室友。性格迥異的他們在同居相處中鬥智鬥勇情況下,<strong>是否會意外觸發心動的代碼?</strong></p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/banner.jpg" alt="banner jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">姜逸城 (邢昭林飾)</h2>
<p class="article-body">28歲,陸漓的學長。性格傲嬌又自戀的霸道總裁。聰明的他是個名副其實的毒舌。喜歡在完全不講道理的情況下講道理。雖然懶散,但他是信奉效率和結果至上主義者,對員工要求極高。後在機緣巧合下成為陸漓的同居室友。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/01.jpg" alt="01 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">陸漓 (祝緒丹飾)</h2>
<p class="article-body">22歲,可愛學霸。暗戀姜逸城,是他同科系的學妹,曾幫他擺平無數次的相親。為了接近他而決定當個女程式設計師,經歷過無數關卡,甚至還女扮男裝,努力進入不接受收女程式設計師的異承公司。後在機緣巧合下成為姜逸城的同居室友。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/02.jpg" alt="02 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">顧筱柒 (關芯飾)</h2>
<p class="article-body">別名小七。陸漓的頭號閨蜜,二次元服裝網店的老闆。熱情活潑、精力充沛。遇到帥哥時,立馬化身為撩漢魔女,但每次戀愛總莫名無疾而終。性格馬虎,常常鬧出笑話來。與顧默是青梅竹馬,乾親至交。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/03.jpg" alt="03 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
</main>
<aside class="sidebar">
<div class="sidebar-widget">
<h2 class="widget-title">導語</h2>
<img src="images/about-love.jpg" alt="about-love jpg" class="widget-image">
<p class="widget-body">
為了自己的暗戀事業和人生目標,陸漓拼盡一切抓住機會,終於和姜逸城達成一年的“契約夫婦”。兩人在同居中展開甜蜜互動,姜逸城對陸漓漸漸動心而不自知……
</p>
</div>
<div class="sidebar-widget">
<h2 class="widget-title">最近文章</h2>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">姜逸城 (邢昭林飾)</h3>
<img src="images/01.jpg" alt="01 jpg" class="widget-image">
</div>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">陸漓 (祝緒丹飾)</h3>
<img src="images/02.jpg" alt="02 jpg" class="widget-image">
</div>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">顧筱柒 (關芯飾)</h3>
<img src="images/03.jpg" alt="03 jpg" class="widget-image">
</div>
</div>
</aside>
</div>
<footer>
<p><strong>非正式官網,網站僅供練習使用。</strong></p>
<p>程序員那麼可愛</p>
</footer>
</body>
</html>
CSS
/* Fonts
-----
font-family: 'Lora', serif;
font-family: 'Ubuntu', sans-serif;
*/
body {
margin: 0;
font-family: 'Ubuntu', sans-serif;
font-size: 1.125rem;
font-weight: 300;
}
img {
max-width: 100%;
display: block;
}
/* =================
Typography
================= */
h1,
h2,
h3 {
font-family: 'Lora', serif;
font-weight: 400;
color: #143774;
margin-top: 0;
}
h1 {
font-size: 2rem;
margin: 0;
}
a {
color: #1792d2;
}
a:hover,
a:focus {
color: #143774;
}
strong {
font-weight: 700;
}
/* h1 subtitle */
.subtitle {
font-weight: 700;
color: #1792d2;
font-size: 0.75rem;
margin: 0;
}
.article-title {
font-size: 1.5rem;
}
.article-read-more,
.article-info {
font-size: 0.875rem;
}
.article-read-more {
color: #1792d2;
text-decoration: none;
font-weight: 700;
}
.article-read-more:hover,
.article-read-more:focus {
color: #143774;
text-decoration: underline;
}
.article-info {
margin: 2em 0;
}
.widget-title,
.widget-recent-post-title {
font-size: 1rem;
}
.widget-title {
font-family: 'Ubuntu', sans-serif;
font-weight: 700;
}
/* =================
Layout
================= */
.container {
width: 90%;
max-width: 900px;
margin: 0 auto;
}
.container-flex {
display: flex;
flex-direction: column;
justify-content: space-between;
}
header {
background: #f8f8f8;
padding: 2em 0;
text-align: center;
margin-bottom: 3em;
}
footer {
background: #143774;
color: #fff;
text-align: center;
padding: 3em 0;
}
@media (min-width: 675px) {
.container-flex {
flex-direction: row;
}
main {
width: 70%;
}
aside {
width: 25%;
min-width: 200px;
margin-left: 1em;
}
}
/* navigation */
nav ul {
list-style: none;
padding: 0;
display: flex;
justify-content: center;
}
nav li {
margin-left: 2em;
}
nav a {
text-decoration: none;
color: #707070;
font-weight: 700;
padding: 0.25em 0;
}
nav a:hover,
nav a:focus {
color: #1792d2;
}
@media (max-width: 675px) {
nav ul {
flex-direction: column;
}
nav li {
margin: 0.5em 0;
}
}
/* articles */
.article-featured {
border-bottom: 1px solid #707070;
padding-bottom: 2em;
margin-bottom: 2em;
}
.article-recent {
display: flex;
flex-direction: column;
margin-bottom: 2em;
}
.article-recent-main {
order: 2;
}
.article-recent-secondary {
order: 1;
}
@media (min-width: 675px) {
.article-recent {
flex-direction: row;
justify-content: space-between;
}
.article-recent-main {
width: 68%;
}
.article-recent-secondary {
width: 30%;
}
.article-featured {
display: flex;
flex-direction: column;
}
.article-image {
order: -2;
min-height: 250px;
object-fit: cover;
/* object-position: left; */
}
.article-info {
order: -1;
}
}
/* widgets */
.sidebar-widget {
border: 20px solid #ebebeb;
margin-bottom: 2em;
padding: 1em;
}
.widget-recent-post {
display: flex;
flex-direction: column;
border-bottom: 1px solid #707070;
margin-bottom: 1em;
}
.widget-recent-post:last-child {
border: 0;
margin: 0;
}
.widget-image {
order: -1;
}
43. Fixing up some loose ends
程式碼範例
HTML – index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>intro to rwd</title>
<link rel="shortcut icon" href="images/cute-programmer.jpg" type="image/x-icon">
<link href="https://fonts.googleapis.com/css2?family=Lora&family=Ubuntu:wght@300;400;700&display=swap" rel="stylesheet">
<link rel="stylesheet" href="css/all.css">
</head>
<body>
<header>
<div class="container container-flex">
<div class="site-title">
<h1>程序員那麼可愛</h1>
<p class="subtitle">Cute Programmer</p>
</div>
<nav>
<ul>
<li><a class="current-page" href="index.html">首頁</a></li>
<li><a href="about-love.html">關於愛情</a></li>
<li><a href="recent-posts.html">劇情介紹</a></li>
</ul>
</nav>
</div> <!-- / .container -->
</header>
<div class="container container-flex">
<main role="main">
<article class="article-featured">
<h2 class="article-title">劇情介紹</h2>
<img src="images/banner.jpg" alt="banner jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
<p class="article-body">陸漓(<a href="#">祝緒丹</a>飾)是女程式設計師。為了追求理想而投身編程領域,她憑藉自身經歷及過人的智慧搞定學長姜逸城(<a href="#">邢昭林</a>飾),擺平他無數次的相親後,成功進入異承公司,勇闖IT世界。倆人因程序代碼結緣,後在機緣巧合下成為同居的室友。性格迥異的他們在同居相處中鬥智鬥勇情況下,<strong>是否會意外觸發心動的代碼?</strong></p>
<a href="#" class="article-read-more">繼續閱讀</a>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">姜逸城 (邢昭林飾)</h2>
<p class="article-body">28歲,陸漓的學長。性格傲嬌又自戀的霸道總裁。聰明的他是個名副其實的毒舌。喜歡在完全不講道理的情況下講道理。雖然懶散,但他是信奉效率和結果至上主義者,對員工要求極高。後在機緣巧合下成為陸漓的同居室友。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/01.jpg" alt="01 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">陸漓 (祝緒丹飾)</h2>
<p class="article-body">22歲,可愛學霸。暗戀姜逸城,是他同科系的學妹,曾幫他擺平無數次的相親。為了接近他而決定當個女程式設計師,經歷過無數關卡,甚至還女扮男裝,努力進入不接受收女程式設計師的異承公司。後在機緣巧合下成為姜逸城的同居室友。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/02.jpg" alt="02 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">顧筱柒 (關芯飾)</h2>
<p class="article-body">別名小七。陸漓的頭號閨蜜,二次元服裝網店的老闆。熱情活潑、精力充沛。遇到帥哥時,立馬化身為撩漢魔女,但每次戀愛總莫名無疾而終。性格馬虎,常常鬧出笑話來。與顧默是青梅竹馬,乾親至交。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/03.jpg" alt="03 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
</main>
<aside class="sidebar">
<div class="sidebar-widget">
<h2 class="widget-title">導語</h2>
<img src="images/about-love.jpg" alt="about-love jpg" class="widget-image">
<p class="widget-body">
為了自己的暗戀事業和人生目標,陸漓拼盡一切抓住機會,終於和姜逸城達成一年的“契約夫婦”。兩人在同居中展開甜蜜互動,姜逸城對陸漓漸漸動心而不自知……
</p>
</div>
<div class="sidebar-widget">
<h2 class="widget-title">最近文章</h2>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">姜逸城 (邢昭林飾)</h3>
<img src="images/01.jpg" alt="01 jpg" class="widget-image">
</div>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">陸漓 (祝緒丹飾)</h3>
<img src="images/02.jpg" alt="02 jpg" class="widget-image">
</div>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">顧筱柒 (關芯飾)</h3>
<img src="images/03.jpg" alt="03 jpg" class="widget-image">
</div>
</div>
</aside>
</div>
<footer>
<p><strong>非正式官網,網站僅供練習使用。</strong></p>
<p>程序員那麼可愛</p>
</footer>
</body>
</html>
HTML – about-love.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>intro to rwd</title>
<link rel="shortcut icon" href="images/cute-programmer.jpg" type="image/x-icon">
<link href="https://fonts.googleapis.com/css2?family=Lora&family=Ubuntu:wght@300;400;700&display=swap" rel="stylesheet">
<link rel="stylesheet" href="css/all.css">
</head>
<body>
<header>
<div class="container container-flex">
<div class="site-title">
<h1>程序員那麼可愛</h1>
<p class="subtitle">Cute Programmer</p>
</div>
<nav>
<ul>
<li><a href="index.html">首頁</a></li>
<li><a class="current-page" href="about-love.html">關於愛情</a></li>
<li><a href="recent-posts.html">劇情介紹</a></li>
</ul>
</nav>
</div> <!-- / .container -->
</header>
<div class="container container-flex">
<main role="main">
<img src="images/about-love.jpg" class="image-full" alt="about-love jpg">
<h2>關於愛情</h2>
<p><strong>為了自己的暗戀事業和人生目標</strong>,陸漓拼盡一切抓住機會,終於和姜逸城達成一年的“契約夫婦”。兩人在同居中展開甜蜜互動,姜逸城對陸漓漸漸動心而不自知……</p>
<p>陸漓(祝緒丹飾)是女程式設計師。為了追求理想而投身編程領域,她憑藉自身經歷及過人的智慧搞定學長姜逸城(邢昭林飾),擺平他無數次的相親後,成功進入異承公司,勇闖IT世界。倆人因程序代碼結緣,後在機緣巧合下成為同居的室友。性格迥異的他們在同居相處中鬥智鬥勇情況下,是否會意外觸發心動的代碼?</p>
<h3>EP01 & EP02</h3>
<p>暗戀就像是等待測試的程序,不確定運行結果,也不確定運行時間,但只要需求沒變,就要努力優化程序,<strong>直到它成功運行</strong>。</p>
<p>喜歡你這件事,不會有任何BUG,所以不需要DEBUG。</p>
<h3>EP03 & EP04</h3>
<p>和暗戀的人在一起時,記得敲一遍高精度,這樣對他的喜歡,就不會溢出,被對方發現了。</p>
<p><strong>每個人都有屬於自己的秘密</strong>,雖然可以通過技術手段進行加密,但卻不知道系統何時會被黑客入侵,將我們保守的秘密公之於眾。</p>
</main>
<aside class="sidebar">
<div class="sidebar-widget">
<h2 class="widget-title">最近文章</h2>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">姜逸城 (邢昭林飾)</h3>
<img src="images/01.jpg" alt="01 jpg" class="widget-image">
</div>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">陸漓 (祝緒丹飾)</h3>
<img src="images/02.jpg" alt="02 jpg" class="widget-image">
</div>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">顧筱柒 (關芯飾)</h3>
<img src="images/03.jpg" alt="03 jpg" class="widget-image">
</div>
</div>
</aside>
</div>
<footer>
<p><strong>非正式官網,網站僅供練習使用。</strong></p>
<p>程序員那麼可愛</p>
</footer>
</body>
</html>
HTML – recent-posts.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>intro to rwd</title>
<link rel="shortcut icon" href="images/cute-programmer.jpg" type="image/x-icon">
<link href="https://fonts.googleapis.com/css2?family=Lora&family=Ubuntu:wght@300;400;700&display=swap" rel="stylesheet">
<link rel="stylesheet" href="css/all.css">
</head>
<body>
<header>
<div class="container container-flex">
<div class="site-title">
<h1>程序員那麼可愛</h1>
<p class="subtitle">Cute Programmer</p>
</div>
<nav>
<ul>
<li><a href="index.html">首頁</a></li>
<li><a href="about-love.html">關於愛情</a></li>
<li><a class="current-page" href="recent-posts.html">劇情介紹</a></li>
</ul>
</nav>
</div> <!-- / .container -->
</header>
<div class="container container-flex">
<main role="main">
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">劇情介紹</h2>
<p class="article-body">陸漓(<a href="#">祝緒丹</a>飾)是女程式設計師。為了追求理想而投身編程領域,她憑藉自身經歷及過人的智慧搞定學長姜逸城(<a href="#">邢昭林</a>飾),擺平他無數次的相親後,成功進入異承公司,勇闖IT世界。倆人因程序代碼結緣,後在機緣巧合下成為同居的室友。性格迥異的他們在同居相處中鬥智鬥勇情況下,<strong>是否會意外觸發心動的代碼?</strong></p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/banner.jpg" alt="banner jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">姜逸城 (邢昭林飾)</h2>
<p class="article-body">28歲,陸漓的學長。性格傲嬌又自戀的霸道總裁。聰明的他是個名副其實的毒舌。喜歡在完全不講道理的情況下講道理。雖然懶散,但他是信奉效率和結果至上主義者,對員工要求極高。後在機緣巧合下成為陸漓的同居室友。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/01.jpg" alt="01 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">陸漓 (祝緒丹飾)</h2>
<p class="article-body">22歲,可愛學霸。暗戀姜逸城,是他同科系的學妹,曾幫他擺平無數次的相親。為了接近他而決定當個女程式設計師,經歷過無數關卡,甚至還女扮男裝,努力進入不接受收女程式設計師的異承公司。後在機緣巧合下成為姜逸城的同居室友。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/02.jpg" alt="02 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
<article class="article-recent">
<div class="article-recent-main">
<h2 class="article-title">顧筱柒 (關芯飾)</h2>
<p class="article-body">別名小七。陸漓的頭號閨蜜,二次元服裝網店的老闆。熱情活潑、精力充沛。遇到帥哥時,立馬化身為撩漢魔女,但每次戀愛總莫名無疾而終。性格馬虎,常常鬧出笑話來。與顧默是青梅竹馬,乾親至交。</p>
<a href="#" class="article-read-more">繼續閱讀</a>
</div>
<div class="article-recent-secondary">
<img src="images/03.jpg" alt="03 jpg" class="article-image">
<p class="article-info">Sep 22, 2021 | 3 comments</p>
</div>
</article>
</main>
<aside class="sidebar">
<div class="sidebar-widget">
<h2 class="widget-title">導語</h2>
<img src="images/about-love.jpg" alt="about-love jpg" class="widget-image">
<p class="widget-body">
為了自己的暗戀事業和人生目標,陸漓拼盡一切抓住機會,終於和姜逸城達成一年的“契約夫婦”。兩人在同居中展開甜蜜互動,姜逸城對陸漓漸漸動心而不自知……
</p>
</div>
<div class="sidebar-widget">
<h2 class="widget-title">最近文章</h2>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">姜逸城 (邢昭林飾)</h3>
<img src="images/01.jpg" alt="01 jpg" class="widget-image">
</div>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">陸漓 (祝緒丹飾)</h3>
<img src="images/02.jpg" alt="02 jpg" class="widget-image">
</div>
<div class="widget-recent-post">
<h3 class="widget-recent-post-title">顧筱柒 (關芯飾)</h3>
<img src="images/03.jpg" alt="03 jpg" class="widget-image">
</div>
</div>
</aside>
</div>
<footer>
<p><strong>非正式官網,網站僅供練習使用。</strong></p>
<p>程序員那麼可愛</p>
</footer>
</body>
</html>
CSS
/* Fonts
-----
font-family: 'Lora', serif;
font-family: 'Ubuntu', sans-serif;
*/
body {
margin: 0;
font-family: 'Ubuntu', sans-serif;
font-size: 1.125rem;
font-weight: 300;
}
img {
max-width: 100%;
display: block;
}
.image-full {
max-height: 300px;
width: 100%;
object-fit: cover;
margin-bottom: 2em;
}
/* =================
Typography
================= */
h1,
h2,
h3 {
font-family: 'Lora', serif;
font-weight: 400;
color: #143774;
margin-top: 0;
}
h1 {
font-size: 2rem;
margin: 0;
}
h3 {
color: #1792d2;
}
a {
color: #1792d2;
}
a:hover,
a:focus {
color: #143774;
}
strong {
font-weight: 700;
}
/* h1 subtitle */
.subtitle {
font-weight: 700;
color: #1792d2;
font-size: 0.75rem;
margin: 0;
}
.article-title {
font-size: 1.5rem;
}
.article-read-more,
.article-info {
font-size: 0.875rem;
}
.article-read-more {
color: #1792d2;
text-decoration: none;
font-weight: 700;
}
.article-read-more:hover,
.article-read-more:focus {
color: #143774;
text-decoration: underline;
}
.article-info {
margin: 2em 0;
}
.widget-title,
.widget-recent-post-title {
font-size: 1rem;
}
.widget-title {
font-family: 'Ubuntu', sans-serif;
font-weight: 700;
}
.widget-recent-post-title {
color: #143774;
}
/* =================
Layout
================= */
.container {
width: 90%;
max-width: 900px;
margin: 0 auto;
}
.container-flex {
display: flex;
flex-direction: column;
justify-content: space-between;
}
header {
background: #f8f8f8;
padding: 2em 0;
text-align: center;
margin-bottom: 3em;
}
footer {
background: #143774;
color: #fff;
text-align: center;
padding: 3em 0;
}
@media (min-width: 675px) {
.container-flex {
flex-direction: row;
}
main {
width: 70%;
}
aside {
width: 25%;
min-width: 200px;
margin-left: 1em;
}
}
/* navigation */
nav ul {
list-style: none;
padding: 0;
display: flex;
justify-content: center;
}
nav li {
margin-left: 2em;
}
nav a {
text-decoration: none;
color: #707070;
font-weight: 700;
padding: 0.25em 0;
}
nav a:hover,
nav a:focus {
color: #1792d2;
}
.current-page {
border-bottom: 1px solid #707070;
}
.current-page:hover {
color: #707070;
}
@media (max-width: 675px) {
nav ul {
flex-direction: column;
}
nav li {
margin: 0.5em 0;
}
}
/* articles */
.article-featured {
border-bottom: 1px solid #707070;
padding-bottom: 2em;
margin-bottom: 2em;
}
.article-recent {
display: flex;
flex-direction: column;
margin-bottom: 2em;
}
.article-recent-main {
order: 2;
}
.article-recent-secondary {
order: 1;
}
@media (min-width: 675px) {
.article-recent {
flex-direction: row;
justify-content: space-between;
}
.article-recent-main {
width: 68%;
}
.article-recent-secondary {
width: 30%;
}
.article-featured {
display: flex;
flex-direction: column;
}
.article-image {
order: -2;
min-height: 250px;
object-fit: cover;
/* object-position: left; */
}
.article-info {
order: -1;
}
}
/* widgets */
.sidebar-widget {
border: 20px solid #ebebeb;
margin-bottom: 2em;
padding: 1em;
}
.widget-recent-post {
display: flex;
flex-direction: column;
border-bottom: 1px solid #707070;
margin-bottom: 1em;
}
.widget-recent-post:last-child {
border: 0;
margin: 0;
}
.widget-image {
order: -1;
}
44. Important Note. The viewport meta tag
The viewport meta
Starting to think responsively
The viewport meta tag
One thing that I don’t include the lessons in this module is the viewport meta tag.
When we take a browser window on our desktop and play with the width, it works well, but it doesn’t fully simulate how a phone will render a page.
The viewport meta tag
To prevent problems form rendering pages that are not optimized for mobile devices and small screens, phones and tablets will actually render a zoomed out version of the page and then shrink it down to fit their viewport.
The viewport meta tag
To prevent it from doing this on our pages, which we’ve optimized for small screens with media queries, we need to add a meta tag to the head of our document.
The viewport meta tag
There are a lot of different attributes that we can add, but the basic meta that you’ll use looks like this:
<meta name="viewport" content="width=device-width, initial-scale=1">
It can be added anywhere in the <head> of your document.
The viewport meta tag
This will ensure that your page actually loads properly on a mobile device, which after all the hard work we did, we really want to do!
So once you’ve graduated from Scrimba and you’re working on your own pages and testing them in different devices, remember to include this in your <head>!
程式碼範例
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>The viewport meta tag</title>
</head>
<body>
</body>
</html>
45. Module wrap up
- You did it!
- Flexbox
- Jusitfy-content & align-items
- flex-direction (switching the main axis)
- Meida queries
- How to build (and style) a navigation
- Pat yourself on the back
- Take a break
- Maybe build the site again!
- Relax and breath a little
- Then it’s time to step up your style!